webpack: Uncaught TypeError: Cannot read property 'call' of undefined
I’m having an issue with webpack where if I stop watching and restart, it will build just fine, but if I edit a file and save it, the incremental build fails with this error:
Uncaught TypeError: Cannot read property ‘call’ of undefined
Hunting it down there’s a module that does not get included in the incremental change build. Best guess is the path followed to re-make the bundle file is not being followed correctly. Upon re-running (stop and start), the missing module is included.
webpack.config.js (module names changed to protect the innocent):
var webpack = require("webpack"),
path = require('path')
;
module.exports = {
entry: {
Entry1: "./app/Entry1.js",
Entry2: "./app/Entry2.js",
Entry3: "./app/Entry3.js",
Entry4: "./app/Entry4.js",
Entry5: "./app/Entry5.js",
Entry6: "./app/Entry6.js",
Entry7: "./app/Entry7.js",
Entry8: "./app/Entry8.js",
},
output: {
path: path.join(__dirname, "public/js"),
filename: "[name].bundle.js",
chunkFilename: "[id].chunk.js"
},
module: {
loaders: [
{test: /\.js$/, loader: 'jsx-loader'}
]
},
plugins: [
new webpack.optimize.CommonsChunkPlugin("shared.js")
]
};
About this issue
- Original URL
- State: closed
- Created 9 years ago
- Reactions: 81
- Comments: 264 (20 by maintainers)
Links to this issue
Commits related to this issue
- Remove CommonsChunkPlugin related code CommonsChunkPlugin がバグってるくさい https://github.com/webpack/webpack/issues/959 しょーがないので window に loader ぶっこむ。 — committed to Whatever-Co/KAMRA-Deja-Vu by Saqoosha 9 years ago
- Hot Module Replacement no longer crashes HMR provided by webpack crashes, with the error: "Uncaught TypeError: Cannot read property 'apply' of undefine" as shown in the following issue on github: htt... — committed to saem/hairpiece by saem 9 years ago
- fix HtmlWebpackPlugin configuration causing this issue : https://github.com/webpack/webpack/issues/959 — committed to 7Kronos/Angular1-scaffold by deleted user 8 years ago
- Stop using DedupePlugin for now Fixes an error in __webpack_require__ when more than one CSS file is required Details in https://github.com/webpack/webpack/issues/959#issuecomment-237438754 — committed to insin/nwb by insin 8 years ago
- Remove webpack's DedupePlugin. DedupePlugin doesn't work correctly. It can cause this exception: "Uncaught TypeError: Cannot read property 'call' of undefined" - https://github.com/webpack/webpack/is... — committed to stefanwille/react-boilerplate by deleted user 8 years ago
- fix(require.ensure): add ArrowFunctionExpression support: Fixes #959 — committed to webpack/webpack by TheLarkInn 8 years ago
- Merge pull request #3544 from webpack/bugfix/add_require_ensure_arrow_function_expression_support fix(require.ensure): add ArrowFunctionExpression support: Fixes #959 — committed to webpack/webpack by sokra 8 years ago
- fix(require.ensure): add ArrowFunctionExpression support: Fixes #959 — committed to nkzawa/webpack by TheLarkInn 8 years ago
- Make common bundles use a hash in their filenames as it might resolve behavior reported in https://github.com/webpack/webpack/issues/959 — committed to kvz/lanyon by kvz 7 years ago
- Correcting HtmlWebpackPlugin option name. The used `chunkSortMode` option should have been `chunksSortMode` (note the extra `s`). Without this, `HtmlWebpackPlugin` may include the common chunks in a... — committed to pvande/multipage-webpack-plugin by pvande 7 years ago
- Correcting HtmlWebpackPlugin option name. (#1) The used `chunkSortMode` option should have been `chunksSortMode` (note the extra `s`). Without this, `HtmlWebpackPlugin` may include the common chunks... — committed to pvande/multipage-webpack-plugin by pvande 7 years ago
- Correcting HtmlWebpackPlugin option name. (#32) The used `chunkSortMode` option should have been `chunksSortMode` (note the extra `s`). Without this, `HtmlWebpackPlugin` may include the common chunk... — committed to zorigitano/multipage-webpack-plugin by pvande 7 years ago
- Fixing up some bugs introduced from webpack switch - Switching bargraph scales from ordinal to ordinal (band) - Adding allChunks param to webpack ExtractTextPlugin Fixes issue with missing module... — committed to JBEI/edd by wmorrell 7 years ago
- :bug: Try to solve webpack issue by adding library name. https://github.com/webpack/webpack/issues/959 — committed to maykinmedia/consumerjs by svenvandescheur 5 years ago
- Stop using DedupePlugin for now Fixes an error in __webpack_require__ when more than one CSS file is required Details in https://github.com/webpack/webpack/issues/959#issuecomment-237438754 — committed to FabianDev1234/nwb by FabianDev1234 8 years ago
Is it really a incorrect bundle/chunk file or is the file in the browser cache just old?
I am also having this issue with v1.12.2
For me the issue goes away if I disable the
DedupePlugin
So, as a temporary solution before this bug has fixed: 1.If you are using both
CommonChunksPlugin
andExtractTextPlugin
, make sure theallChunks
option ofExtractTextPlugin
is set totrue
; 2.If you are usingHtmlWebpackPlugin
, set optionschunksSortMode
to'dependency'
3.If you did all operations before, trouble is still alive, try solve the dependencies by your hand.(change the order you import the scripts in html)Please fix the issue~
In case it’s any help to others:
My issue was that the ExtractTextPlugin was used in production. By default, the plugin only extracts the text of the initially-loaded chunks. Fixing it, for me, was as simple as adding
allChunks: true
like so:@sokra dang, this error is so annoying, it disrupts the workflow a lot. Not every time but often. I am both using multiple entry points and the commonChunkPlugin. Is this error going to gt fixed, I am not sure on how to help.
Pretty please have another look at this bug!
Happened to me also. Learned that it’s a cache issue on the server. Users where getting outdated chunks. I’ve fixed this with adding hash (
[chunkhash]
) to chunks, so the setting I’ve updated is :chunkFilename: '[id].chunk.[chunkhash].js',
.Hope this helps someone.
pls kill me
Sorry, I should have mentioned how I fixed it. If you look here https://github.com/AngularClass/angular2-webpack-starter/blob/b305c9a8071465ee0817a59093c9f73fd1cc7278/config/webpack.common.js#L240...L243 the chunk order is now correct via
chunksSortMode: 'dependency'
which will ensure this orderpolyfills, vendor, main
which means the webpack IIFE is included in polyfills which is the first script to loadIt’s interesting to see such a prominent bug seems to last forever in webpack. I’ve ditched javascript and switched to multi-platform app development using Python (the Kivy framework) for one year now and I’ve become a much happier man:)
Still, have this problem. with producation building
Adding
chunksSortMode: 'dependency'
to theHtmlWebpackPlugin
fixed it for me, thanks @gdi2290 !Same problem here, problem occurs after watch has detected a change and builds the bundle.
v1.11.0
We’ll look into this. Def seems like a problem.
I’m seeing the same issue with the latest webpack and
optimization.splitChunks
on.immortal bug 😛
I’m still seeing this issue. Using the latest version v1.10.1
First build works fine, but incremental builds fail. I get the following error from my bundle:
Uncaught TypeError: Cannot read property 'apply' of undefined
It seems to break on the usage of
apply
in this function.The hashes of the builds are different.
After a little trial’n’error I narrowed this bug down to being a caching issue. If you add
cache: false
it does build fine. Just that it is slow. Still a better than re-starting manually.I hope this helps fixing the bug. Isn’t it a saying that the two most difficult things in programming are
cache invalidation
and …This issue seems to go away after removing the Dedupe plugin. Related issue: https://github.com/webpack/webpack/issues/583
Remove the
DedupePlugin
and all work right (;@TheLarkInn @sokra @jhnns @SpaceK33z
Is there anything that can be done around here that would make this issue easier to diagnose? Better error message? More context in the error message?
It feels every time we make a half significant change to our webpack configuration (adding/moving/removing plugins/optimisataions) we end up hitting this and have a hard time working out where the problem is sourced from since it does not have a single root problem. Given the amount of comments on this issue I’d say other people feel this pain too.
it still exists in webpack@4.21.0
setting
concatenateModules: false
in optimization fixed the problem in my case@papermana Were you using arrow functions in your
require.ensure()
?Btw I’m using
webpack@2.2.0-rc.1
Just came across this issue myself with the following extremely simple code:
I stepped back and thought “well to simplify this even further, let’s not use a fancy arrow here” and I changed it to this:
…voila…it magically worked.
At first I assumed that this had to do with the fact that arrow functions use lexical
this
binding especially since many of the errors here revolve around.call(..)
and.apply(..)
. I dug in a little further though and set some breakpoints in DevTools around the failing area.I’m no webpack source expert (yet) so bare with my source illiteracy but it seems that every
moduleId
passed into the below function should be a number.All is well when using a regular function as the callback to
require.ensure()
however when using an arrow function I noticed eventually themoduleId
will end up being a string.This is where things failed seemingly because
installedModules[moduleId]
would returnundefined
. So what I did is on the fly replacedmoduleId
with2
(in my current case that was the correctmoduleId
of my async module).Finally when we set
module = installedModule[moduleId]
,module
was a valid object and notundefined
.TL;DR
There seems (?) to be some issue with
moduleId
’s resolved value when using an arrow function inrequire.ensure(..)
if my analysis is right?cc: @TheLarkInn
同问,还是有这个问题。为什么问题关闭?
Nvm I have a reproducible example. New tests passing fix should coming up shortly
I’m facing the same issue with
DedupePlugin
. I’m using webpack 2 beta 20 and named Commons chunks -For everyone running into this, The problem is usually the the order if the files and webpack’s module wrap when you use CommonsChunkPlugin or HtmlWebpackPlugin
for HtmlWebpackPlugin you can manually sort your files with
chunksSortMode
for CommonsChunkPlugin the order of the when webpack creates chunks matter since they inject
webpackJsonp
in the main file. Here’s a simple pseudocode example (may not work)main.js
vendor.js
In this pseudocode example of webpack, you can see how the order of when the files are loaded matters a lot since
webpackJsonp
is defined in themain
file whilereact
andreact-dom
are defined invendor.js
If you are using both
CommonChunksPlugin
andExtractTextPlugin
, make sure theallChunks
option ofExtractTextPlugin
is set totrue
; this seemed to be the fix for me.See https://webpack.js.org/plugins/extract-text-webpack-plugin/#options (specifically the
allChunks
section)I removed
webpack.optimize.OccurrenceOrderPlugin
and it became fine again.Had the same issue without using the
DedupePlugin
.I got
Uncaught TypeError: Cannot read property 'call' of undefined
inmanifest.js
.It was pretty simple after I found the solution: I had to add
chunksSortMode: 'dependency'
to myHtmlWebpackPlugin
because without it the index.html resulted in script tags in the wrong order (the vendor.js was the last one).it also happens on v1.12.2.
In my case it happens in an entry point which is not included in the
CommonsChunkPlugin
as a chunk. If I remove theCommonsChunkPlugin
it works fine.However, I do have a few more entry points that are not included in the
CommonsChunkPlugin
and they work fine. The only thing that is special with this specific entry file is that the required files are not really modules. They don’t export anything. This entry point is for the marketing site of the project and it just concatenates a few scripts so it looks like this:and these files have a few jquery stuff with no use of
module.exports
Re-open please?
I’m also getting this problem, using webpack 4.31.0 and SplitChunksPlugin. I’ve provided a minimal project setup that reproduces the problem: https://gitlab.com/jlapointe/codesplittingtest
I’ve tried most of the fixes suggested earlier in the thread (toggling various config fields, including concatenateModules), but no luck.
Some commonalities with previous comments:
'../../fixtures/mock_users.json'
, similar to https://github.com/webpack/webpack/issues/959#issuecomment-118394128I’m having the error
webpack 2.2.1
In my case, I’m creating a commons chunk from 2 entry points, each representing an app. These apps both
import
the same module via code-splitting. The error is caused when trying toeval
animport 'graphiql/graphiql.css';
in the module in question.~I’ve noticed that other modules
import
ed via code-splitting only in one of the apps don’t have this problem. Moreover, I can see in the output of weback that the corresponding CSS of the working modules are properly extracted by the ExtractTextPlugin whereas the CSS of the failed module are not.~EDIT: all modules which import css or scss have the error in question.
EDIT 2: I’m using
allChunks: true
for my ExtractTextPlugin and the issue is gone. This however means that the CSS is not delivered via code-splitting but it’s build during compile time with all the other assets. I found some issues in the ExtractTextPlugin repo that perhaps are related to this.sokra:
https://gitter.im/webpack/webpack/archives/2015/02/04
Was able to fix it with a combination of chunk hashing and webpack route prefetching. The prefetching logic prevents the client from requesting outdated chunks, and the chunk hashing forces the chunks to update once new ones are available server side. With only one or the other, chunks either crash or fail to update. I believe this is because we have code splitting implemented in our react appRoot.
I would also like to note the often proposed solution of adding
chunksSortMode: 'dependency'
completely broke our update cycle and prevented the app from properly updating at all. I dont recommend it for production code.My fix: (Webpack 4.46.0 & react 16.14.0 with HtmlWebpackPlugin)
chunkFilename: '[id].chunk.[chunkhash].js',
as proposed by @nicolaelitvacimport(/* webpackPrefetch: true */ './path/to/SettingsPage.js');
Note: This solution only worked perfectly after the second app update as the prefetching logic must be live on the client to prevent loading outdated chunks.
Edit: This did not entirely fix our issue and the problem persists 😦
never ending…
My experience: I faced this error 2 times:
contenthash
infixed by adding
I had this issue and it turns out it was because I had 2 chrome windows open with my app. I did some changes on my app, then refreshed one of those windows to see the changes and it threw this error, and it kept showing until I closed the other window, then again refresh my window and it worked. Hope this helps someone.
@louisscruz thanks, i fixing it with your method. Just adding
allChunks: true
as so:If you’re using hard-source-webpack-plugin or babel-loader with the cacheDirectory option enabled, try deleting the contents of the cache directory; this defaults to
node_modules/.cache/**
. For me, this fixed the issue.Also running into this bug, but only in production:
Running 2.1.0-beta18, with Aurelia rc update. Using 3 separate chunks (aurelia-bootstrap, aurelia, app), <script loaded in the right order (production == dev).
Removing the common-chunks plugin and just using a single entrypoint seems to fix it.
@foffer you add
debug: true
to thewebpack.config.js
same level asentry
andplugins
. Not within the CommonsChunkPlugin. Also note this is just a workaround that makes it less anoying, but you will now have to wait for all files to re-build. So it is far from a solution. The only thing you get out of it is that you do not manually have to re-start webpack after every change.@airwin I am not using the
DedupePlugin
in development but the bug is there. So it is not caused by theDedupePlugin
only.Here people working with angular2 fight with this random wild, very dengerous error: https://github.com/AngularClass/angular2-webpack-starter/issues/456
The same
Uncaught TypeError: Cannot read property 'call' of undefined
bug on webpack 1.12.9.It breaks on this line in the runtime (I’ve extract the runtime in a separate chunk, and inserted it into the html):
As @zbyte64 noted, disabling the DedupePlugin plugin fixes the problem.
To reproduce:
Then serve the content in the
build/
directory and see the bug:😭I’m still seeing this issue. Using the latest version v1.11.0
To fix this issue, just try every enabled plugins in production mode, which means changing property values in
optimization
field. The fields includeAnd most of the time, disable
removeEmptyChunks
,mergeDuplicateChunks
,occurrenceOrder
andconcatenateModules
will help.I had this problem suddenly, and the only thing I did was change
devtool
to'source-map'
in the development webpack config.Everything was fine before that, but my working copy was broken no matter what I did. Even though I reverted the change, I kept getting the erro. I’ve tried: 1) changing setting back, 2) stashing working copy changes, 3) deleting node_modules, 4)
git clean -dxf
, all to no avail.I tried reverting back to before we added
CommonsChunkPlugin
, and it was working then, and then I checked outHEAD
again, and it wasn’t working. Removing@babel/polyfill
as @louisscruz mentioned resolved the issue, however we need the polyfills.Further investigation resulted in noticing that requiring
css-loader
is where the error is coming from, so I moved ourimport '...application.scss'
to aboveimport 'babel-polyfill'
… and that worked.No idea why my working copy got busted, nor why it was working for the last week but suddenly not now (I had restarted Webpack multiple times in there so it wasn’t a hanging configuration). Seems to be a load order issue of some kind related to assets, at least for us.
In my case I also have error 😢 but I have webpack 3.11.0
HashedModuleIdsPlugin is enabled, and my CommonsChunkPlugins settings is
Entry:
On picture you can see what moduleId is 0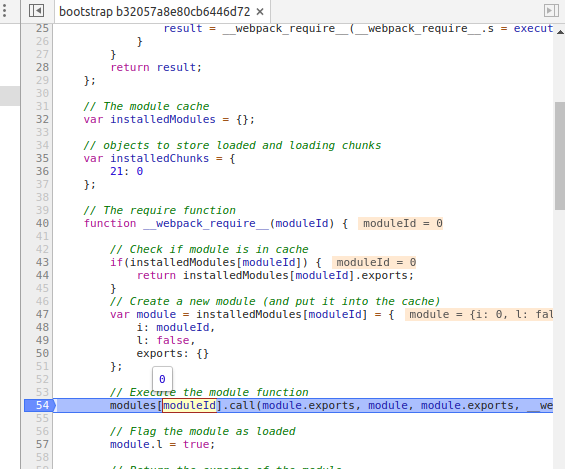
I have reproduced it. I am using Angular-CLI version 1.6.5 in order to build very simple Angular app. I have attached .zip file with the project and instructions in README.md. Here is how you can reproduce the error:
Sample package.json file:
Please see attached file for reproduction. It also contains webpack build in
dist
folder that causes the error.Edit: Forgot to mention my observation - if I perform
ng build --prod --sourcemaps
the error disappears…Edit 2: Forgot to mention also that if you switch off angular build optimizer, the error will disappear but the size of your bundle will increase -
ng build --prod --build-optimizer=false
call-error-repro.zip
I’m was having same issue using ExtractCssChunks. Only working when the project was rebuilt. Resolved this using allChunks: true
I was just running into this, for me it was the ModuleConcatenationPlugin plugin.
@TheLarkInn which version will fix this problem ?
I changed
CommonsChunkPlugin.minChunks
toInfinity
, the problem is gone, but thevendors.js
has nothing.From
DedupePlugin
https://webpack.github.io/docs/list-of-plugins.html#dedupeplugin :I got it solved by removing it from my development config
Same here. Get this error when using the
CommonsChunkPlugin
with an optionalminChunks
function. Somehow it causes the main (entry) file inclusion as dependent one into the common (vendor.js in my case) chunk and then its removal after optimization (or somethign like) from so that it leads to the runtime error once absent module is called. Have no ideas why it happens but resolution for me is removing any conditions from theminChunks function
that might accidentally specify the entry file name as a target to the commons chunks list. Then it works well.Im having this issue also, it seems to be caused by the named chunks in the CommonsChunkPlugin.
If I comment out the chunks line, it works as expected
Edit: I was eventually able to fix the problem by removing the angular chunk (which didnt need to be there) Still not 100% on the problem though.
Why is this closed? This is still an issue
Awesome, it works. https://webpack.js.org/configuration/optimization/#optimizationconcatenatemodules
I’m getting this change too for webpack v4.10.2. No code change, updated package lock and
production
is broken.I was stuck with this using 1.13.2 and 1.14.0. It came up when I added a second instance of the CommonsChunkPlugin. Adding
minChunks: 1
solved the issue. No idea why.I have the same promble
Uncaught TypeError: Cannot read property 'call' of undefined
. I think the reason maybe if you use code spliting, webpack will generate a id for the chunk. Every build time, the chunk id maybe change, but if you don’t change the chunk file, this file’s chunkhash will not change, so the browser will use the cache first. This time, the another file which require the chunk by chunk id will get the error chunk id.@sickboy Thanks a lot for the comment. We at @taskworld are experiencing this problem on production, and your comment helped me investigate the problem further.
I think I found the root problem:
We’re using long-term caching,
DedupePlugin replaces duplicated module with another module’s number, but that number isn’t used to hash the chunk.
Reproducing the problem
webpack.config.js builds src/main1.js and src/main2.js into separate build folders.
src/a.js
src/b.js
src/c.js is a duplicate of src/a.js
src/d.js is a duplicate of src/b.js
src/main1.js
src/main2.js
The case of src/main1
In the case of main1,
./a
is required before./b
, so:./a
gets ID 1./b
gets ID 2When it gets built, the chunk file 8dd6ff7e4b0954b12f3f.bundle.js looks like this:
The case of src/main2
In the case of main1,
./b
is required before./a
, so:./a
gets ID 2./b
gets ID 1When it gets built, the chunk file 8dd6ff7e4b0954b12f3f.bundle.js looks like this:
The hash is the same as previous run, which breaks long-term caching.
Proposed solution
When replacing the module with another module’s ID, webpack should hash the module by another module’s ID instead of its content.
I faced this issue today. In my case, I had to change this syntax:
into this:
@sickboy This problems happened to me with 2.1.0-beta20。When i debugged the source code in Chrome-devTools , I find this:
module 0 , 1 must be external jquery
Cannot read property ‘call’ of undefined
I don’t want to remove the common chunks and use a single entrypoint…
I also had this issue.
I upgraded from 1.12.14 to 1.13.0 without relief.
I removed my use of CommonsChunkPlugin and was promptly relieved (thanks @stream7, @orrybaram, @hinell). However, my bundle sizes increased.
I put back the CommonsChunkPlugin and added cache: false. Was again relieved (thanks @janusch), but my build time had increased.
Then I realized I had two separate “webpack --watch” processes running on the source at the same time. Aha! That could mess with caching, couldn’t it?
Ceasing to “webpack --watch” twice relieved me of this issue. In my boneheaded case, I no longer needed cache: false and could safely use the CommonsChunkPlugin. Small bundles, fast builds: workflow restored.
follow step by step this solution for fix this problem, working with me using webpack 4
1.STEP ONE output: { library: ‘[name]’, umdNamedDefine: false }
optimization: { concatenateModules: false, providedExports: false, usedExports: false }
STEP TWO remove symlink and caheWithContenxt
STEP THREE remove react hot module or disable this for production
STEP FOUR remove old project name in heroku, after remove old project create new project name
Still, have this problem. why issue closed?
try
sudo ionic serve
or in windows open shell as Administratorthis is beause of the “use strict” , in ES5, arguments.callee() and arguments.caller() are forbid , you can delete the file “.babelrc " ->” ‘modules’: false " , everything is OK.
the only thing i wanna know is how this problems happened
i am getting the same error. local it works fine but in prod it fails randomly.
my webpack.config.js seems like this
new CommonsChunkPlugin({ “name”: “inline”, “minChunks”: null }), new CommonsChunkPlugin({ “name”: “vendor”, “minChunks”: (module) => module.resource && module.resource.startsWith(nodeModules), “chunks”: [ “main” ] }),
pls help me where i need to make changes
I was experiencing this issue and unfortunately, none of the solutions above helped me.
I created the After Chunk Hash Plugin to alleviate the issue.
It essentially waits until all assets have been output, and then rehashes them based on their final contents - it’s a bit of a hack, but I’ve been using it in production for the last few months on a large scale build & site, and it’s been working great.
It works with:
manifest.json
from Webpack Manifest Plugin (or similar plugins)Full info as to what it solved has been posted in the readme
Same issue here, but I found a fix!
First, I want to explain that I have a
vendor
entry that looks like this:Before (broken)
My strategy has been to have a
vendor.js
file with all thenode_modules
and all the other common chunks go in acommon.js
file. Works great for a production build, but fails on the dev server with “Uncaught TypeError: Cannot read property ‘call’ of undefined”.The
moduleId
is not found, probably because I picked anynode_modules
out of an existingcommon
chunk.After (fixed)
The solution was to treat the dev server differently and only do a
vendor.js
file that has not only all thenode_modules
, but also all the common chunks, all in one file.Hope this helps someone!
just remove ‘new webpack.optimize.CommonsChunkPlugin’
I’m on 2.1.0-beta.25. I have tried all the tips above, and the only one that ‘works’, is to set minChunks to Infinity in the CommonsChunkPlugin. Removing DedupePlugin made no difference for me. I see in chrome that it is looking for module id 2, but as you can see from the array, that number is skipped.
When I run webpack --display-modules I get the same thing, nr 2 is missing 😃
I then created a stats.json file and analyzed it in https://webpack.github.io/analyse. Everything was fine.
When I search in stats.json I see that id:2 is jQuery , which I have defined as external.
Is there a change in how externals work in webpack 2? By the way, this partitcular problem - call undefined - disappears if i remove the externals section.
Thomas
Maybe it’s fixed… Please reopen if still an issue with 1.8.4
I had the same problem with this error message. I removed a empty style tag in my newly added component that cause the issue:
After that it worked again!
Hi everyone,
I just fix this issue by using this webpack.mix.js configuration :
I’m using :
The point to change is at publicPath. By default chunkFilename will save the file at public folder on Laravel framework, as long as we are not put folder name before the chunkFilename. example : js/[id].chunk.[chunkhash].js will automatically save the file on public/js/ folder if we dont put any folder name before the chunkFilename, all the file will save on public folder on Laravel.
So laravel will find the generated chunkFilename from public folder. There is a different path between using laravel on our local PC and on the server
If we run Laravel on our local PC, we usually use command php artisan serve. And if we want to call a file inside public folder, we don’t need to put public on url when you call a file. example : http://localhost:8080/app.js
but at the server we need to put public folder. example : https://yoursite.com/public/app.js
That’s why i set publicPath: ‘/public/’ to tell the laravel that my chunkFilename generated inside public folder
I don’t need to downgrade my laravel-mix. Hope this help anyone that still have the problem
@apertureless I am using version 3. But version 4 also works if I remove the
<style></style>
tags from the components.In my case, I was getting the error because I was attempting to import files already packaged by webpack for production but I didn’t package them properly. The “properly” part required that I specify:
@strickland84 I think, in laravel’s
webpack.mix.js
:Or in
webpack.config.js
:But this didn’t work for me…
@nicolaelitvac thanks for the above - where did you set this config? Which file? Thanks
This happens to me a lot as well. I’m working on an Angular Monorepo with internally built angular libraries. When we make changes to the libraries, we use a --watch flag, which triggers an incremental build, then the main app does the same, every time the build either fails, or it succeeds, but any action aftewards results in this error until I restart the app (via webpack a la ng serve command)
EDIT: I’m happy to provide whatever details I can to resolve the issue, just need someone from the webpack team to let me know what they need from me and I will produce it for them.
change my minChunkSize fixed
It happened to me from nowhere. I was happily compiling styled Vue single file components. Now if the component has a
<style>
tag the compilation fails with this very error. Removing the style fixes the compilation.after some tests I decided that my build breaks on config:
i simply stop the serving and npm start again this solves for me.
I believe that I might have found a reproduceable scenario to recreate this issue consistently. It occurs when 1 named entry point imports another named entry point. For example:
This scenario will cause the
Component.js
exported in a way that causes this error.I just had the same issue of “call of undefine” … i was able to resolve it by downgrading to version 4.9.2 which is a more stable version… it worked… however … the issue returned when i added cacheGroups not set to default: false…
cacheGroups: { vendors: { test: /[\/]node_modules[\/]/, priority: -10 }
when it is set to default: false … the issue is gone…
I have just run into this using Webpack 4.12.0
For me it seems that it only exhibits itself when
I tried production mode with
devtool: 'eval'
,plugins: [new webpack.NamedModulesPlugin()]
andoptimization: {minimize: false}
and it still failed so I’m confident its got nothing to do with the module naming/ids or minification.The entry point which contains no unique code contains something like this in the runtime code
deferredModules.push([null,9]);
which is where the error seems to come from as the null value is obviously not an actual module id. It should be something likedeferredModules.push([462,9]);
My workaround was to create an small shim file which doesn’t actually do anything but import the actual entry file and export it again. Then I set the entry point to this shim file. e.g.
The root cause is what @gdi2290 said. Because “You must load the generated chunk before the entry point” CommonsChunkPlugin
In my case, I used [‘common’, ‘c1’, ‘c2’] in HtmlWebpackPlugin, I used
I don’t know why it works before. So I change “chunk1.id - chunk2.id” to “chunk2.id - chunk1.id”, all chunks are in right order now! Problem fixed.
@WouterVanherck I only experienced the ‘call’ of undefined error as a result of upgrading to Webpack 4. The only other thing I did was make sure that the plugins I’d added to my Webpack configuration were upgraded to versions compatible with Webpack 4.
Looking at your stack trace and https://github.com/webpack/webpack/blob/master/lib/TemplatedPathPlugin.js it seems like you’re probably not on the latest version of that plugin. Just a guess though.
Removing CommonChunksPlugin worked for now
This was happening to me because I accidentally overwrote a bundle with the same name:
fooBar
in my webpack.configimport(/* webpackChunkName: "fooBar" */ "./index")
Changing it to
import(/* webpackChunkName: "fooBarLazy" */ "./index")
fixed my issue (as would removing the chunkname entirely).I was still getting some issues in my production builds, however this workaround solved it for me: https://github.com/AnujRNair/webpack-after-chunk-hash-plugin After some small adjustments, it seems to work well for my situation. (See the open issues for my two adjustments).
Im also trying out https://github.com/adventure-yunfei/md5-hash-webpack-plugin as an alternative.
add
Ok I think I got it working. The issue always appears in webpack 2.3.0 and up, but (apparently) it works on version 2.2.0.
I’m only having this issue on Cloud9 IDE, by the way. On my local machine it works fine.
There’s nothing particular about my
CommonChunkPlugin
:Anything new I could try?
Which version fixes this issue? Still seeing this issue with Webpack 2.2.0
Edit: Turned out to be a caching issue
I’m going to tackle this right now, if anyone can provide a very small reproducible example, that would be excellent. I should have enough information to build test cases that should fail, but it would be extra helpful if possible 🙏
DedupePlugin broke our production build. After removing it, our build is fine. Webpack version is 2.1.0-beta.25, used in the context of react-boilerplate (https://github.com/mxstbr/react-boilerplate).
A “npm install react-boostrap” was enough to trigger the problem.
Removing dedupe plugin solved this for me as well. The problem started when I added
NamedModulesPlugin
. My project has three css imports and dedupe plus extract-text-plugin meant that the first two modules ended up like"./node_modules/typeface-alegreya/index.css": "./styles/zenburn.css"
which apparently Webpack can’t follow when doing module resolution. I’m on Webpack 1.I am not using
HtmlWebpackPlugin
and of all the suggestions, the one that worked was not to use theDedupePlugin
.Unfortunately this results into a significantly bigger bundle (in terms of size).
Any other ideas on how to resolve this?
@sokra Is this a “core” Webpack thing or something related to “the plugins”?
Apologies this was on accident.
When I first got this error I “fixed” it by removing the
DedupePlugin
but after a while it appeared again. Since I don’t use theHtmlWebpackPlugin
I tried out a few different things and managed to work around it by removing theUglifyJsPlugin
.I just run into this bug; in my case, the problem was using pure ES6. As soon as I transpiled it with Babel, the problem went away. Just a note.
HI All
Just for information, I founf that this error it is a bit misleading, it is not a bug but the way how you build your common chunks. please refer to
this works for me
https://github.com/webpack/webpack/tree/master/examples/multiple-commons-chunks or https://github.com/webpack/webpack/tree/master/examples/common-chunk-and-vendor-chunk
Found a similar issue in our production builds. I could only reproduce it by setting NODE_ENV to “production”. Removing
DedupePlugin
from our webpack config file did the trick, but now our bundle sizes are bigger. Please fix!! I’d be more than happy to provide more info.We’re using webpack@1.12.15
@janusch where do you add that option, under CommonsChunkPlugin?
+1 Have same issue when run karma in watch mode. Workaround remove entry property of webpack config object in karma.conf.js
Same problem here.
Quite difficult to understand exactly how to reproduce the problem, but it seems to happen more frequently when I edit a symlinked module or simply a module inside
node_modules
during a watch build.v1.12.1
I’m facing this issue only when I use ExtractTextPlugin + SplitByPathPlugin (or split by name). It goes away if I remove either one of them.