vetur: Cannot find module xxx
Info
- Platform: Windows Server 2016
- Vetur version:
- VS Code version:
Problem
The error message “cannot find module ‘components/models’” is displayed within vscode although the file is clearly there… When running the code with tsc
no error is displayed.
Reproducible Case
Simply create a new Quasar project by running quasar create <project>
as described here.
Additional info
Following the FAQ I tried to fix it but no luck. The things I’ve tried are:
- All TS settings within Vetur:
- Enable Vetur: Use Workspace Dependencies
- Reinstall the Vetur extension
Files
models.ts
export interface Todo {
id: number;
content: string;
}
export interface Meta {
totalCount: number;
}
CompositionComponent.vue
<template>
<div>
<p>{{ title }}</p>
<ul>
<li v-for="todo in todos" :key="todo.id" @click="increment">
{{ todo.id }} - {{ todo.content }}
</li>
</ul>
<p>Count: {{ todoCount }} / {{ meta.totalCount }}</p>
<p>Active: {{ active ? 'yes' : 'no' }}</p>
<p>Clicks on todos: {{ clickCount }}</p>
</div>
</template>
<script lang="ts">
import { defineComponent, PropType, computed, ref } from '@vue/composition-api';
import { Todo, Meta } from './models';
function useClickCount() {
const clickCount = ref(0);
function increment() {
clickCount.value += 1
return clickCount.value;
}
return { clickCount, increment };
}
function useDisplayTodo(todos: Todo[]) {
const todoCount = computed(() => todos.length);
return { todoCount };
}
export default defineComponent({
name: 'CompositionComponent',
props: {
title: {
type: String,
required: true
},
todos: {
type: (Array as unknown) as PropType<Todo[]>,
default: () => []
},
meta: {
type: (Object as unknown) as PropType<Meta>,
required: true
},
active: {
type: Boolean
}
},
setup({ todos }) {
return { ...useClickCount(), ...useDisplayTodo(todos) };
}
});
</script>
Config
@quasar/app/tsconfig-preset/tsconfig-preset.json
{
"compilerOptions": {
"allowJs": true,
// `baseUrl` must be placed on the extending configuration in devland, or paths won't be recognized
"esModuleInterop": true,
"module": "esnext",
"moduleResolution": "node",
// Needed to address https://github.com/quasarframework/app-extension-typescript/issues/36
"noEmit": true,
"resolveJsonModule": true,
"sourceMap": true,
"strict": true,
"target": "es6",
// Quasar-defined webpack aliases
"paths": {
"src/*": ["src/*"],
"app/*": ["*"],
"components/*": ["src/components/*"],
"layouts/*": ["src/layouts/*"],
"pages/*": ["src/pages/*"],
"assets/*": ["src/assets/*"],
"boot/*": ["src/boot/*"]
},
// Forces quasar typings to be included, even if they aren't referenced directly
// Removing this would break `quasar/wrappers` imports if `quasar`
// isn't referenced anywhere, because those typings are declared
// into `@quasar/app` which is imported by `quasar` typings
"types": ["quasar"]
},
// Needed to avoid files copied into 'dist' folder (eg. a `.d.ts` file inside `src-ssr` folder)
// to be evaluated by TS when their original files has been updated
"exclude": ["/dist", ".quasar", "node_modules"]
}
ts.config.json
{
"extends": "@quasar/app/tsconfig-preset",
"compilerOptions": {
"baseUrl": ".",
"target": "es5",
}
}
Thank you for your help.
About this issue
- Original URL
- State: closed
- Created 4 years ago
- Reactions: 1
- Comments: 29 (5 by maintainers)
Please follow #2377
@
=./src/
maybe this is problem
Make sure to use workspace version of TS in VSCode so that it loads local TS plugins. Refer to this comment: https://github.com/vitejs/vite/issues/965#issuecomment-717248892
With VSCode, you can select your workspace TS Version:
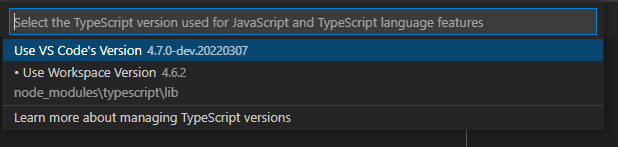
I think my issue is related to 890, .424 and 815.
To whom it may concern.
In my case I had a completely decouple Vue app inside my Laravel app in
/public/MyVueApp/
and I experienced the same problem as OP (albeit for a different reason).All I had to do is open the Vue app root folder as a new project in VSCode, and everything worked fine after that.
In the Quasar Framwework default aliases
src
is an alias for/src
:The
src
webpack alias works perfectly fine but is not recognized by Vetur. So I blieve the issue lies within Vetur? The only import that is not underlined by Vetur is the last one, which is not a webpack alias but a relative path:It seems like Vetur does not resolve the WebPack alias.