apollo: Cannot use apollo in Typescript @Component decorator with 'components' key
In my vue typescript application, I’m trying to use vue apollo with the vue-property-decorator
library.
I have the following code:
import { Component, Prop, Vue } from 'vue-property-decorator'
import HeaderPrimary from '@/components/Header/HeaderPrimary.vue'
import query from '@/graphql/users.gql'
@Component({
components: { // Error from here
HeaderPrimary, // ...
}, // to here.
apollo: {
users: query,
},
})
export default class TheHeader extends Vue {
users: any = null
}
I’m receiving the following error:
Argument of type '{ components: { HeaderPrimary: {}; }; apollo: { users: DocumentNode; }; }' is not assignable to parameter of type 'VueClass<Vue>'.
Object literal may only specify known properties, but 'components' does not exist in type 'VueClass<Vue>'. Did you mean to write 'component'?
When I remove the apollo
part of the @Component
, the error goes away.
The app still runs fine and queries correctly, but unfortunately I’m not sure how to get past this TypeScript error.
About this issue
- Original URL
- State: open
- Created 6 years ago
- Reactions: 3
- Comments: 16 (5 by maintainers)
I had the same type error problem and looked at the typings for the
VueApolloComponentOptions
. It seems the query should be either aDocumentNode
or a function returning aDocumentNode
, but for some reason, it errors on theDocumentNode
alone.Your error should be sorted out with
Just add
"vue-apollo"
intsconfig.json
:@Acidic9 @jurgisrudaks @gdpaulmil
I suggest this is not gonna be happened if vue-apollo module is imported correct. If it’s not loaded, it could be like as @Acidic9 said. like below
but if you import vue-apollo module somewhere on your codebase, it should be work. As you can see error has disapieared.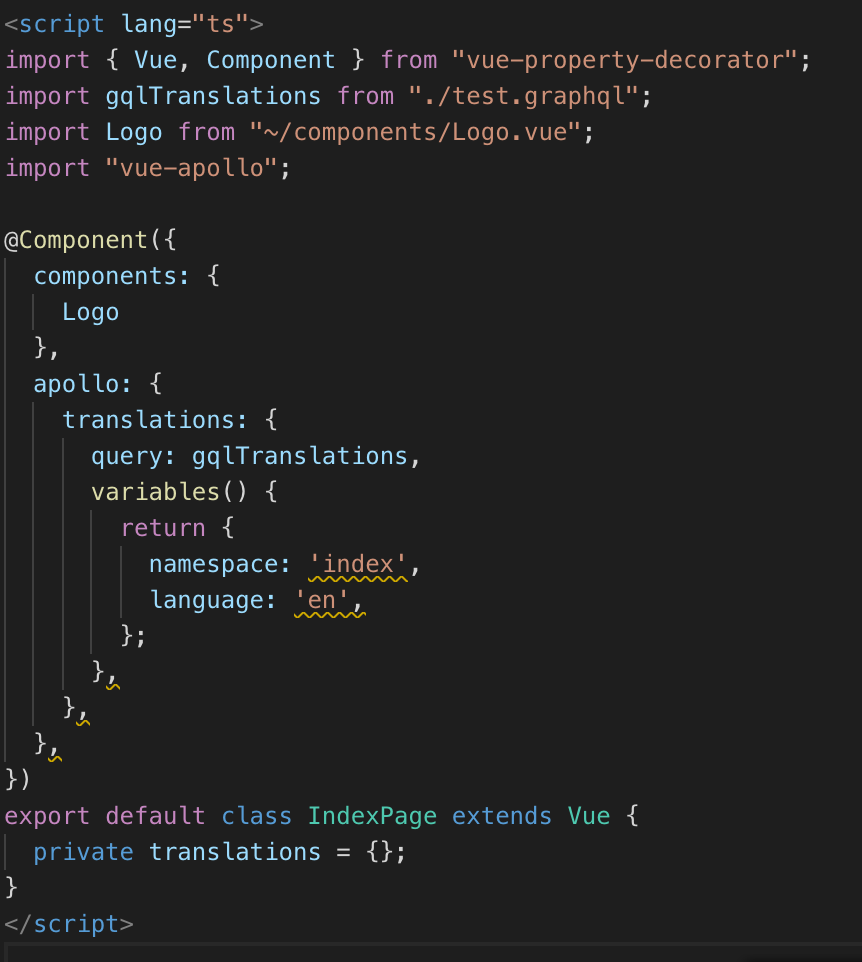
I have exactly the same issue and unfortunately @Kresten suggestion didn’t work for me. Any help would be much appreciated 😃
Source:
index.vue
translations.gql
See https://v4.apollo.vuejs.org/guide-composable/
That doesn’t work for me. I’ve imported vue-apollo as shown and in multiple other places. The only situation that works for a
DocumentNode
is assigning with a function: