ts-node: Cannot find module defs using typeRoots
If you have a config like this:
{
"compilerOptions": {
...
"typeRoots" : ["./typings"]
}
And a directory structure like this:
-- tsconfig.json
-- typings
-- index.d.ts
ts-node will not be able to find the module defs in index.d.ts
ts-node will work if you have something like:
-- tsconfig.json
-- typings
-- <library_name>
-- index.d.ts
Typescript will work with either setup.
About this issue
- Original URL
- State: closed
- Created 6 years ago
- Reactions: 2
- Comments: 15 (11 by maintainers)
@blakeembrey Can you be more specific? I have read the README several times over and the only workaround I have been able to find is the one @shusson mentioned, which is to break my module declarations out into
index.d.ts
files in folders named after the module and add atypeRoots
section.My
tsconfig.json
does not have a reference tofiles
,include
orexclude
, in fact it is quite small/simple:The readme says:
Great, I am not using any of those compiler options so that doesn’t apply to me.
It then goes on to say:
Great, I have some global module definitions so I can put them into
typeRoots
.Unfortunately, this is where things fall apart, putting my module definitions into a file in
typeRoots
does not work as I expect.I somewhat suspect (after reading the readme 3 more times while drafting this response) that the magic is in this statement:
If I’m interpreting that correctly, when combined with your insistence that “the answer is in the README”, it is saying that the
typeRoots
mentioned previously only works if the types are setup as type package folders within thetypeRoots
directory?Since I don’t want to be yet another person who complains but offers no solutions, I would recommend rewording that section to be more clear. Looking through GitHub issues, there are about a dozen issues with people struggling with this same problem, which suggests to me that the way it is currently worded is not immediately apparent to the casual ts-node user.
Perhaps something like:
What part of my configuration is causing this to happen? I have included my
tsconfig.json
, and in facttsc
works without thetypeRoots
section at all. Is there some other config you are referring to besidestsconfig.json
? I’m just runningtsc
in the directory with thetsconfig.json
file and it is successfully compiling, I am not passing in any additional compiler options or providing any files.@blakeembrey did something change here? This does not work for us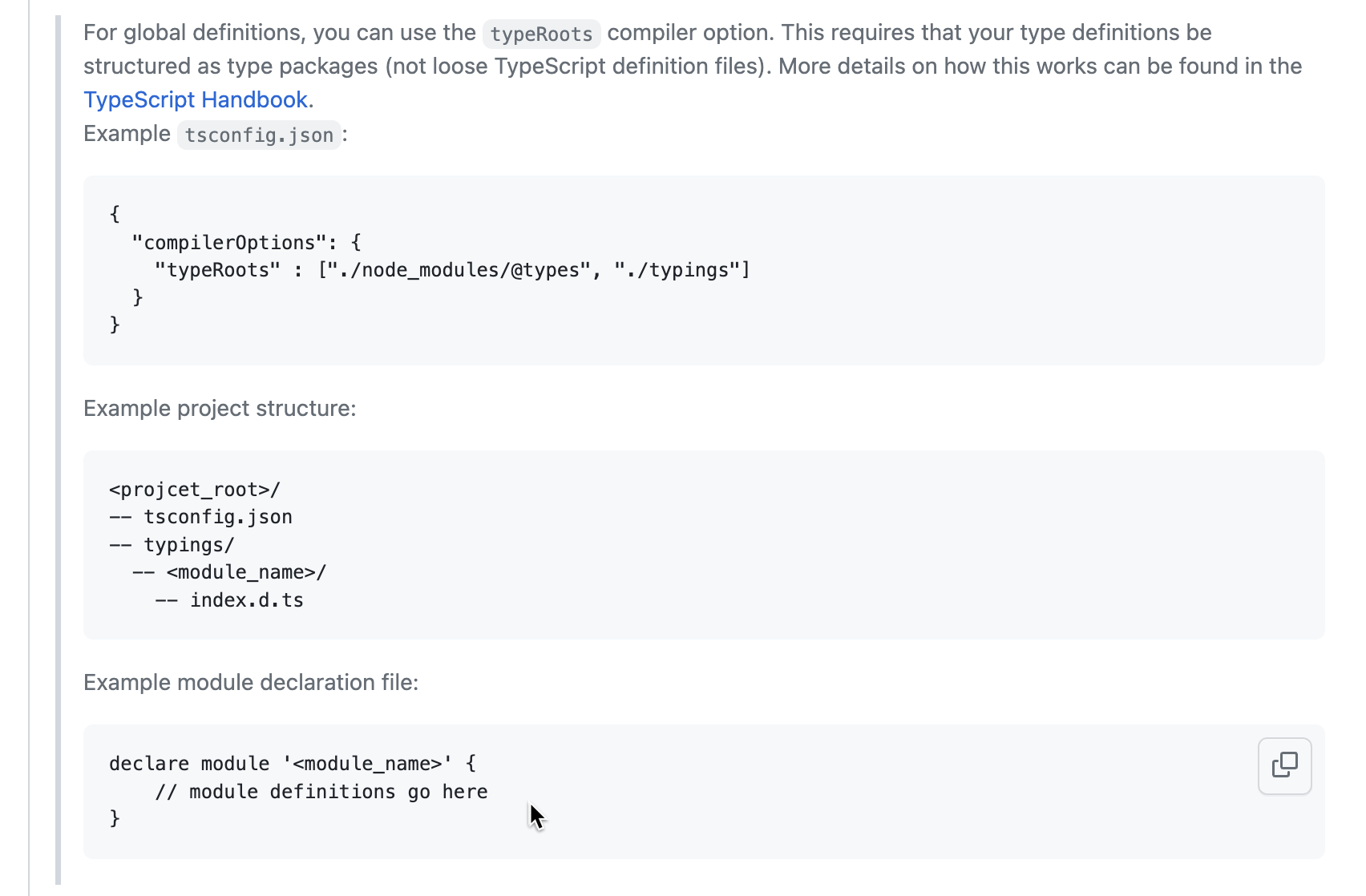
We have to configure all modules in the paths instead
we use
Ok @blakeembrey , but then
ts-node
should have worked with with the--files
flag right?PR to edit documentation to hopefully reduce the number of people that show up here in the issues with the same problem: https://github.com/TypeStrong/ts-node/pull/698
Can you provide some details on why this is not the correct structure? It is accepted by TypeScript compiler without problem. There are two supported (as far as I know) ways to have modules. One is by creating some
.d.ts
file intypeRoots
directory (e.g.,my.d.ts
) with one or moredeclare module 'whatever' { ... }
blocks in it, and another is to create a a folder namedmyModule
with a file namedindex.d.ts
in it that containsdeclare module 'myModule' { ... }
. Both methods appear to be fully supported by the compiler, but only the latter method appears to be supported by ts-node.At the least, the README should be updated to mention that you cannot use the former style, and ideally a brief blurb or link to the reasoning would be appreciated.
See the README. This is not the correct structure. It most likely works with TypeScript because you have it explicitly or explicitly in your includes.