react-native-reanimated: RN 0.61: Animated.color causes "excessive number of pending callbacks"
Hey guys, how are you?
Just updated react native version to 0.61 (rc) and now my app is crashing on some screens that use color interpolation.
We have a theme based template, so I created a function that parses hex colors using polished:
import Animated from 'react-native-reanimated'
import { parseToRgb } from 'polished'
const interpolateColors = (animation, { inputRange, outputRange }) => {
const colors = outputRange.map(parseToRgb)
const r = Animated.interpolate(animation, {
inputRange,
outputRange: colors.map(c => c.red),
extrapolate: Animated.Extrapolate.CLAMP,
})
const g = Animated.interpolate(animation, {
inputRange,
outputRange: colors.map(c => c.green),
extrapolate: Animated.Extrapolate.CLAMP,
})
const b = Animated.interpolate(animation, {
inputRange,
outputRange: colors.map(c => c.blue),
extrapolate: Animated.Extrapolate.CLAMP,
})
return Animated.color(r, g, b)
Any tips on this?
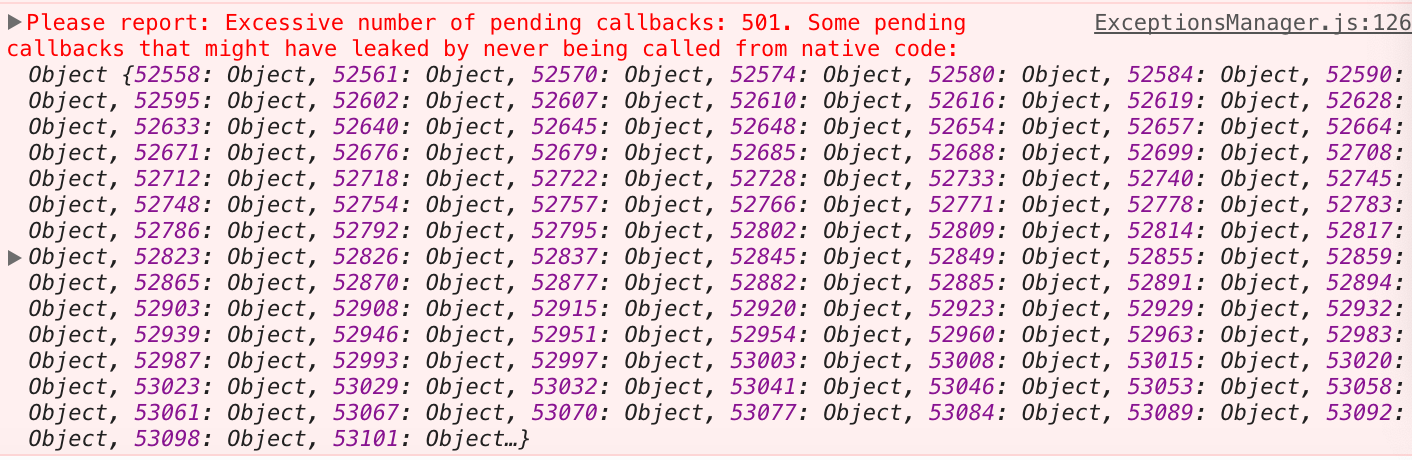
Thanks!
About this issue
- Original URL
- State: closed
- Created 5 years ago
- Reactions: 20
- Comments: 22 (4 by maintainers)
We just landed a PR to core that changes this warning to only fire once the first time it occurs. That might also make this issue less high severity.
I get the same error in some conditions using react-navigation 5.0.0 (unstable)
But now after some time on it I suspect that I know where your issue is coming from.
There is only one place in reanimated where we use RN’s callbacks and it is when the animated node detaches. If you have more than 500 nodes that unmount at the same time we’d enqueue an equal number of calls with callbacks (we use callback on detach to remember the last value of animated node in JS so that if it is being reused we initiate it in native with that value). The relevant code in reanimated is here https://github.com/kmagiera/react-native-reanimated/blob/c841c34d1037e6b00a9f425e17782ccfbdb92a12/src/core/InternalAnimatedValue.js#L25 – I’ll try to think how we can workaround this limitation (maybe we could use events, or maybe detect which nodes are not going to be reused such that we only use callbacks on the ones that will)