react-native-reanimated: "Attempting to assign to readonly property" error when using SharedValue across Runtimes (VisionCamera) (interpolateColor: `getInterpolateCacheRGBA`)
Description
Iām using this hook:
export function useAnimatedColor(
color: Reanimated.SharedValue<string>,
): Readonly<Reanimated.SharedValue<string | number>> {
const animation = useSharedValue(0);
const colorFrom = useSharedValue(DEFAULT_COLOR);
const colorTo = useSharedValue(color.value);
useAnimatedReaction(
() => color.value,
(newColor, prevColor) => {
animation.value = 0;
colorFrom.value = prevColor ?? DEFAULT_COLOR;
colorTo.value = newColor;
animation.value = withTiming(1, {
duration: 150,
easing: Easing.linear,
});
console.log(`Animating from ${prevColor} -> ${newColor}`);
},
);
// TODO: Using colorFrom and colorTo in here raises "Attempting to assign to readonly property" error...
return useDerivedValue(() =>
interpolateColor(animation.value, [0, 1], [colorFrom.value, colorTo.value]),
);
}
which results in this error being thrown:
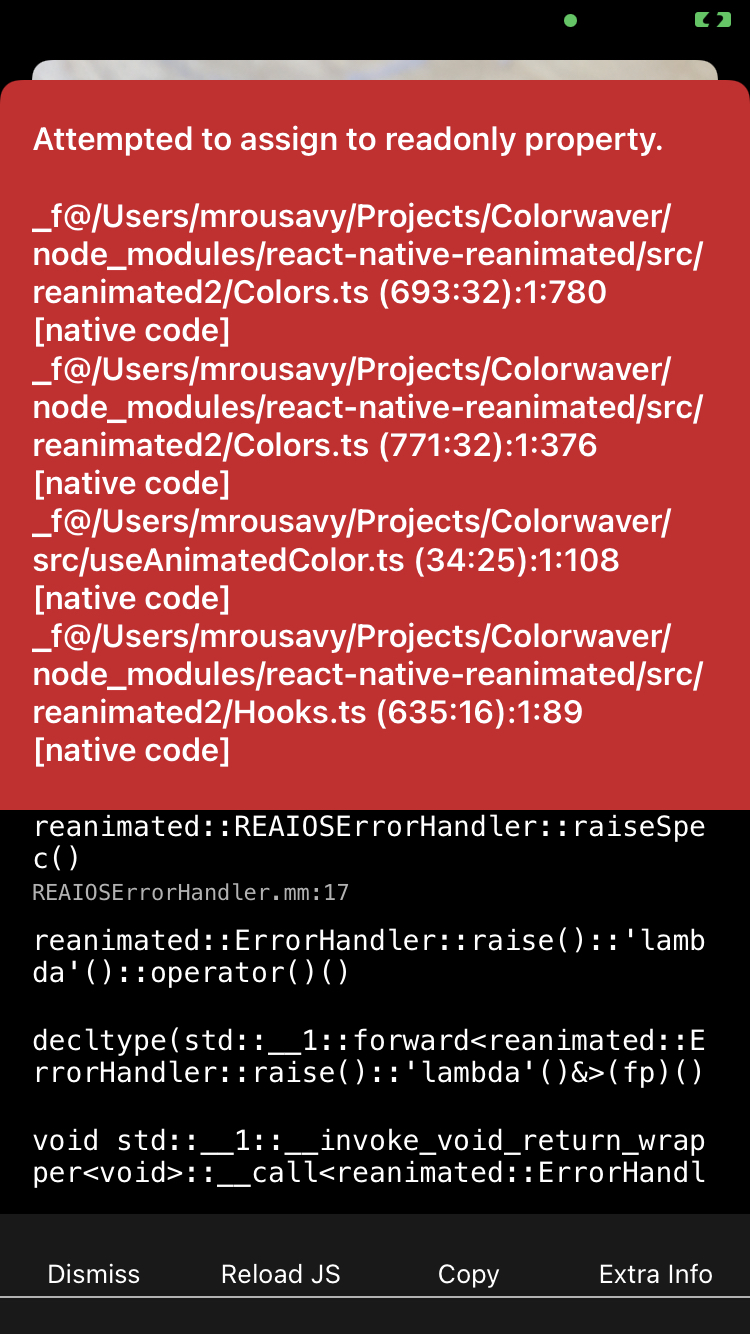
Expected behavior
I expect it to interpolate correctly
Actual behavior & steps to reproduce
It crashes
Snack or minimal code example
https://github.com/mrousavy/Colorwaver (this code throws the error. the passed in SharedValue is being assigned here)
Package versions
- React Native: 0.65.1
- React Native Reanimated: 2.3.0-alpha.2
- NodeJS: 14
- Xcode: /
- Java & Gradle: /
Affected platforms
- Android (not tested)
- iOS
- Web (not tested)
About this issue
- Original URL
- State: closed
- Created 3 years ago
- Reactions: 8
- Comments: 17 (5 by maintainers)
Commits related to this issue
- Patch Reanimated for Cache "assign" bug Issue: https://github.com/software-mansion/react-native-reanimated/issues/2329 — committed to mrousavy/Colorwaver by mrousavy 3 years ago
- Redesign (v2) (#6) * Add fastlane * Add CI workflows * Update ids * Enable Hermes * Revert "Enable Hermes" This reverts commit 780e1db6726add52863f8f7c2e160989f8fffca6. * Update Gem... — committed to mrousavy/Colorwaver by mrousavy 3 years ago
- add patch for reanimated bug the patch is a fix for https://github.com/software-mansion/react-native-reanimated/issues/2329 — committed to goaliath-app/goaliath by OliverLSanz 2 years ago
- Fix immutable color cache (#2796) ## Description The color cache was mutable only from the JS side. I decided to remove the cache and instead of this I created a new faster function with a better ... — committed to software-mansion/react-native-reanimated by piaskowyk 2 years ago
- Fix immutable color cache (#2796) ## Description The color cache was mutable only from the JS side. I decided to remove the cache and instead of this I created a new faster function with a better ... — committed to aeddi/react-native-reanimated by piaskowyk 2 years ago
This is fixed with reanimated 2.2.4 btw, which also works with rn 0.66.0
(Or this issue was never introduced in that minor version to begin with š )
Yep, suspicion confirmed. If I remove the caching completely, everything runs smoothly:
I got the same error on iOS using
react-native-reanimated@2.3.0-beta.3
andreact-native@0.66.1
.I got rid of the error by enabling hermes in the Podfile and running
npx pod-install