react-monaco-editor: Not working with nextjs?
Describe the bug i try to use react-monaco-editor with next.js based on this tutorial. But all time i have a lot of errors:
Uncaught (in promise) Error: Unexpected usage
at EditorSimpleWorker.push../node_modules/monaco-editor/esm/vs/editor/common/services/editorSimpleWorker.js.EditorSimpleWorker.loadForeignModule (editorSimpleWorker.js:540)
at webWorker.js:54
Uncaught TypeError: Failed to construct 'URL': Invalid URL
at push../node_modules/normalize-url/index.js.module.exports (index.js:46)
at getReloadUrl (hotModuleReplacement.js:74)
at hotModuleReplacement.js:92
at NodeList.forEach (<anonymous>)
at reloadStyle (hotModuleReplacement.js:89)
at update (hotModuleReplacement.js:119)
at invokeFunc (debounce.js:95)
at trailingEdge (debounce.js:144)
at timerExpired (debounce.js:132)
Uncaught (in promise) Error: Unexpected usage
at EditorSimpleWorker.push../node_modules/monaco-editor/esm/vs/editor/common/services/editorSimpleWorker.js.EditorSimpleWorker.loadForeignModule (editorSimpleWorker.js:540)
at webWorker.js:54
my code:
import React from 'react'
import PropTypes from 'prop-types'
import * as monaco from '@timkendrick/monaco-editor/dist/external'
import MonacoEditor from 'react-monaco-editor'
window.MonacoEnvironment = { baseUrl: '/monaco-editor-external' }
/**
* @param {string} code
* @returns {*}
* @constructor
*/
const CodeEditor = ({code}) => {
const options = {
selectOnLineNumbers: true
}
return (
<MonacoEditor
// width="800"
height="600"
language="javascript"
theme="vs"
// theme="vs-dark"
value={''}
options={options}
onChange={() => {}}
editorDidMount={() => {}}
/>
)
}
CodeEditor.propTypes = {
code: PropTypes.string.isRequired,
}
export default CodeEditor
in server.js added:
...
server.use(
'/monaco-editor-external',
express.static(`${__dirname}/node_modules/@timkendrick/monaco-editor/dist/external`)
)
...
To Reproduce install “react-monaco-editor”: “^0.34.0” “monaco-editor”: “^0.20.0” “monaco-editor-webpack-plugin”: “^1.9.0” “@timkendrick/monaco-editor”: “0.0.9” “next”: “^9.2.1” “react”: “^16.12.0”
Expected behavior work without errors
Environment (please complete the following information):
- OS: mac
- Browser chrome
- Bundler webpack
guys, please, help me. I spent for that a lot of time, i tried use with/without MonacoWebpackPlugin
, with/without @stoplight/monaco, but i still got error 😦
About this issue
- Original URL
- State: open
- Created 4 years ago
- Reactions: 4
- Comments: 27
Commits related to this issue
- test: lazy loaded editors do not have the monaco worker loaded. refer to monaco-editor loading in: https://github.com/react-monaco-editor/react-monaco-editor/issues/271#issuecomment-601816334 — committed to influxdata/ui by wiedld 2 years ago
- test: lazy loaded editors do not have the monaco worker loaded. refer to monaco-editor loading in: https://github.com/react-monaco-editor/react-monaco-editor/issues/271#issuecomment-601816334 — committed to influxdata/ui by wiedld 2 years ago
- test: lazy loaded editors do not have the monaco worker loaded. We need monaco window object for language registration, then link monaco workers to react-monaco-editor. refer to: https://github.com/... — committed to influxdata/ui by wiedld 2 years ago
- test: lazy loaded editors do not have the monaco worker loaded. We need monaco window object for language registration, then link monaco workers to react-monaco-editor. refer to: https://github.com/... — committed to influxdata/ui by wiedld 2 years ago
- test: lazy loaded editors do not have the monaco worker loaded. We need monaco window object for language registration, then link monaco workers to react-monaco-editor. refer to: https://github.com/... — committed to influxdata/ui by wiedld 2 years ago
- test: lazy loaded editors do not have the monaco worker loaded. We need monaco window object for language registration, then link monaco workers to react-monaco-editor. refer to: https://github.com/... — committed to influxdata/ui by wiedld 2 years ago
- test: lazy loaded editors do not have the monaco worker loaded. We need monaco window object for language registration, then link monaco workers to react-monaco-editor. refer to: https://github.com/... — committed to influxdata/ui by wiedld 2 years ago
The following is what worked for me.
package.json
some typescript file
example usage
@subwaymatch thx, you saved my day!
@monaco-editor/react just works out of the box, no need to mess up with next.js config
Ok figured it out 😄
next.js.config
:Now, in the
index.js
page file:If you read all the way down, turns out
worker-loader
did nothing, so you can remove that.They key is to set the
MonacoWebpackPlugin
filename
key to have thestatic
prefix, so it’s added to the.next/static
build output, which is then exposed via a HTTP request under_next/static/
.Happy coding!
I’ve spent a few days trying to make
react-monaco-editor
with next.js. It just didn’t work 😩. While next.js has an example withreact-monaco-editor
in the official repo, it breaks as soon as I add Tailwind or sass. Even if I do get it to work with the tutorial here, I don’t like the idea of polluting the Webpack config (and having to install extra packages).A much, much better alternative IMHO is to use @monaco-editor/react. I’m using css modules with sass, and it works like magic (especially after spending a few days with this package). I also didn’t have to fiddle with
next.config.js
. For those who may be interested, there’s a simplified CodeSandbox example with Next.js by the author of that package.Screenshot of how I’m using it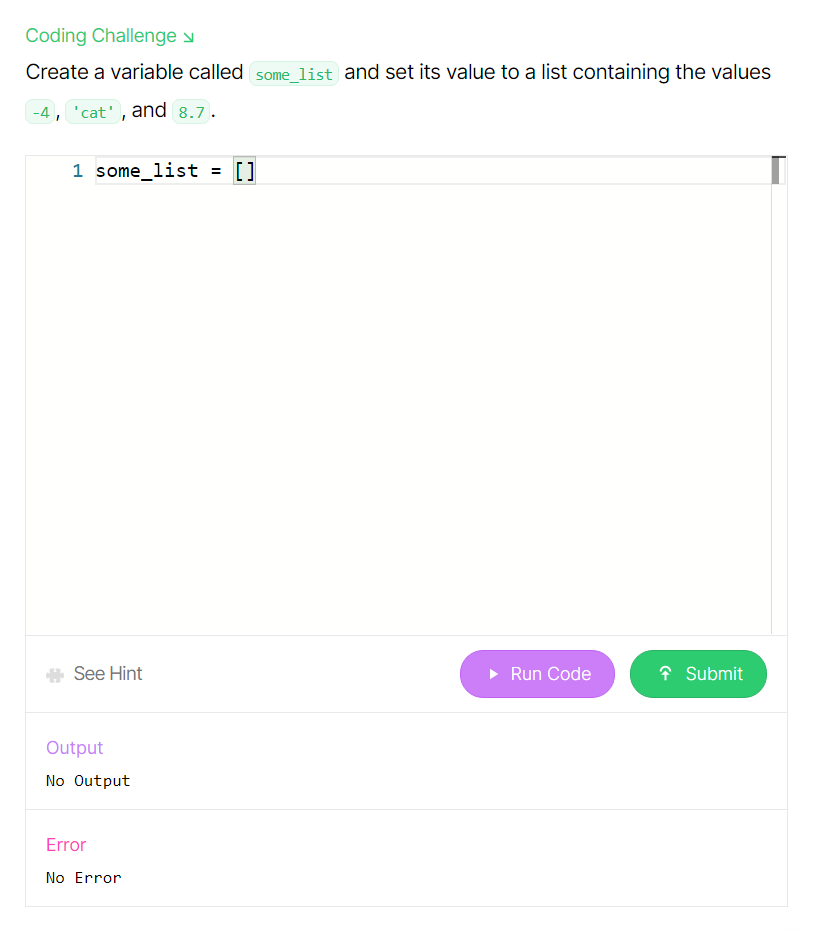
After some experiments, I got it working with the help of next-transpile-modules.
Also, make sure to import the editor dynamically and disable SSR:
Also, make sure you only have
react-monaco-editor
and not(!)monaco-editor
in your dependencies (not sure, but otherwise, it didn’t work for me).By the way, the reason why I don’t like to use
@monaco-editor/react
is that I don’t want to depend on an external CDN from where the workers are downloaded from.I hope this helps someone.
If you check the console while you type it will probably spit out a load of errors, which are related to the service worker not running
By default Monaco just tries to load the works from a base url like site.com/ts.worker.js
That doesn’t exist with next js, so you have to provide Monaco with the paths which next exposes them as
Trying to get this working myself, has been rather painful so far, lots of Googling & reading…
So far I have it working with
javascript
syntax, but using saytypescript
throws errors about the service workers not working. Putting this here to document where I have got to for others:First off, you need to install the CSS & font loader plugins for NextJS:
yarn add @zeit/next-css next-fonts
This allows the css which monaco imports internally to be parsed, and also the fonts they bundle/load to be parsed:
Next up, I added the webpack plugin. If I leave the languages out it complains (even though the docs suggest it defaults to them all?):
Then I had some errors about the service works not being found… so I added the
worker-loader
:yarn add worker-loader
I added the rules to parse any of the “[language].worker.js” files:
Now back in my pages file, I import the module. It doesn’t seem to be compatible with SSR, so I just load it on the client (don’t think this is a big issue anyway, who wants code to be indexed?):
So this currently works, with the worker seemingly doing it’s job highlighting in the background. If the worker isn’t loaded, there is a lot of lag when typing:
Of course, i’m aware of that. turns out i’ve installed
@monaco-editor/react
but did not installedmonaco-editor
and the error throws irrelevantModule not found: Can't resolve '@monaco-editor/react'
. Thanks for the reply anyway! good dayNot working for me.
@AlbinoGeek Thank you soo much for the simple working example!
What are you using to compile the code ? :3
I’ve followed @Ehesp to change the
next.config.js
andindex.js
, and now it shows the error message…Any solution ?😭
nice work @Ehesp ! do you mind explaining a little why you need to “override the worker URL that monaco uses to load the workers”? it’s not clear why you had to do that. i tried your steps without it and it still worked. was a little laggy at the start and then became pretty good. was it purely a perf improvement thing?