puppeteer: Puppeteer 1.10 stops after creating browser context on Linux
Steps to reproduce
Tell us about your environment:
- Puppeteer version: 1.10.0
- Platform / OS version: Linux / Ubuntu 16.04.4 (within Docker)
- URLs (if applicable): n/a
- Node.js version: 8.12
What steps will reproduce the problem?
Please include code that reproduces the issue.
#!/usr/bin/env node
'use strict';
const puppeteer = require('puppeteer');
console.log('loaded puppeteer');
(async () => {
const browser = await puppeteer.launch();
console.log('launched puppeteer');
console.log(await browser.userAgent());
console.log(await browser.version());
console.log(await browser.wsEndpoint());
console.log(await process.versions);
browser.on('targetcreated', () => console.log('+++ Browser target created'));
const context = await browser.createIncognitoBrowserContext();
console.log('created context');
const page = await context.newPage();
console.log('created page');
await page.goto('https://example.com');
console.log('loaded page');
console.log('length:'+(await page.content()).length);
console.log('got content');
await browser.close();
console.log('finished');
})();
What is the expected result? On Mac with same version of Puppeteer 1.10.0:
loaded puppeteer
launched puppeteer
Mozilla/5.0 (Macintosh; Intel Mac OS X 10_14_1) AppleWebKit/537.36 (KHTML, like Gecko) HeadlessChrome/72.0.3582.0 Safari/537.36
HeadlessChrome/72.0.3582.0
ws://127.0.0.1:60441/devtools/browser/5c9660e4-36e0-4e55-a14e-723b6aad3724
{ http_parser: '2.8.0',
node: '8.11.3',
v8: '6.2.414.54',
uv: '1.19.1',
zlib: '1.2.11',
ares: '1.10.1-DEV',
modules: '57',
nghttp2: '1.32.0',
napi: '3',
openssl: '1.0.2o',
icu: '60.1',
unicode: '10.0',
cldr: '32.0',
tz: '2017c' }
created context
+++ Browser target created
created page
loaded page
length:1262
got content
finished
On Linux with previous version of Puppeteer 1.9.0:
loaded puppeteer
launched puppeteer
Mozilla/5.0 (X11; Linux x86_64) AppleWebKit/537.36 (KHTML, like Gecko) HeadlessChrome/71.0.3563.0 Safari/537.36
HeadlessChrome/71.0.3563.0
ws://127.0.0.1:34785/devtools/browser/c6332edc-dbc3-4399-bf93-be582cf32d86
{ http_parser: '2.8.0',
node: '8.12.0',
v8: '6.2.414.66',
uv: '1.19.2',
zlib: '1.2.11',
ares: '1.10.1-DEV',
modules: '57',
nghttp2: '1.32.0',
napi: '3',
openssl: '1.0.2p',
icu: '60.1',
unicode: '10.0',
cldr: '32.0',
tz: '2017c' }
created context
+++ Browser target created
created page
loaded page
length:1262
got content
finished
What happens instead? On same Linux with Puppeteer 1.10.0:
loaded puppeteer
launched puppeteer
Mozilla/5.0 (X11; Linux x86_64) AppleWebKit/537.36 (KHTML, like Gecko) HeadlessChrome/72.0.3582.0 Safari/537.36
HeadlessChrome/72.0.3582.0
ws://127.0.0.1:36749/devtools/browser/a4b48e44-25c5-47af-b6a9-848bf7327bfb
{ http_parser: '2.8.0',
node: '8.12.0',
v8: '6.2.414.66',
uv: '1.19.2',
zlib: '1.2.11',
ares: '1.10.1-DEV',
modules: '57',
nghttp2: '1.32.0',
napi: '3',
openssl: '1.0.2p',
icu: '60.1',
unicode: '10.0',
cldr: '32.0',
tz: '2017c' }
created context
+++ Browser target created
and then hangs with Chrome still running in the background. No other output.
Launching puppeteer with dumpio provides the following:
launched puppeteer
Mozilla/5.0 (X11; Linux x86_64) AppleWebKit/537.36 (KHTML, like Gecko) HeadlessChrome/72.0.3582.0 Safari/537.36
HeadlessChrome/72.0.3582.0
ws://127.0.0.1:38707/devtools/browser/4d0c4fd3-e30d-4d1a-ba9a-cd077b567c90
{ http_parser: '2.8.0',
node: '8.12.0',
v8: '6.2.414.66',
uv: '1.19.2',
zlib: '1.2.11',
ares: '1.10.1-DEV',
modules: '57',
nghttp2: '1.32.0',
napi: '3',
openssl: '1.0.2p',
icu: '60.1',
unicode: '10.0',
cldr: '32.0',
tz: '2017c' }
created context
[1106/144733.934456:FATAL:proc_util.cc(97)] Check failed: fstatat(proc_self_fd, de->d_name, &s, 0) == 0.
#0 0x55b883ccd7df <unknown>
#1 0x55b883c46f70 <unknown>
#2 0x55b88510b223 <unknown>
#3 0x55b885109424 <unknown>
#4 0x55b885107480 <unknown>
#5 0x55b887b17865 <unknown>
#6 0x55b887b1745f <unknown>
#7 0x55b8838a9b07 <unknown>
#8 0x55b8838aac8d <unknown>
#9 0x55b8838dc42b <unknown>
#10 0x55b8838a9111 <unknown>
#11 0x55b887c68488 <unknown>
#12 0x55b887c6832e <unknown>
#13 0x55b8838d96b5 <unknown>
#14 0x55b8819f11ac <unknown>
#15 0x7f60ab39c830 __libc_start_main
#16 0x55b8819f102a <unknown>
Received signal 6
#0 0x55b883ccd7df <unknown>
#1 0x55b883ccd351 <unknown>
#2 0x7f60b1514390 <unknown>
#3 0x7f60ab3b1428 gsignal
#4 0x7f60ab3b302a abort
#5 0x55b883ccc185 <unknown>
#6 0x55b883c473e2 <unknown>
#7 0x55b88510b223 <unknown>
#8 0x55b885109424 <unknown>
#9 0x55b885107480 <unknown>
#10 0x55b887b17865 content::RendererMainPlatformDelegate::EnableSandbox()
#11 0x55b887b1745f <unknown>
#12 0x55b8838a9b07 <unknown>
#13 0x55b8838aac8d msE
#14 0x55b8838dc42b <unknown>
#15 0x55b8838a9111 <unknown>
#16 0x55b887c68488 <unknown>
#17 0x55b887c6832e <unknown>
#18 0x55b8838d96b5 <unknown>
#19 0x55b8819f11ac <unknown>
#20 0x7f60ab39c830 __libc_start_main
#21 0x55b8819f102a <unknown>
r8: 00007f60ab742770 r9: 00007f60b1a8fac0 r10: 0000000000000008 r11: 0000000000000202
r12: 00007ffdff4261a0 r13: 0000000000000069 r14: 00007ffdff4261a8 r15: 00007ffdff4261b0
di: 0000000000000001 si: 0000000000000001 bp: 00007ffdff425760 bx: 00007ffdff4257c0
dx: 0000000000000006 ax: 0000000000000000 cx: 00007f60ab3b1428 sp: 00007ffdff425628
ip: 00007f60ab3b1428 efl: 0000000000000202 cgf: 002b000000000033 erf: 0000000000000000
trp: 0000000000000000 msk: 0000000000000000 cr2: 0000000000000000
[end of stack trace]
Calling _exit(1). Core file will not be generated.
+++ Browser target created
[1106/144733.904805:FATAL:proc_util.cc(97)] Check failed: fstatat(proc_self_fd, de->d_name, &s, 0) == 0.
#0 0x55b883ccd7df base::debug::StackTrace::StackTrace()
#1 0x55b883c46f70 logging::LogMessage::~LogMessage()
#2 0x55b88510b223 sandbox::ProcUtil::HasOpenDirectory()
#3 0x55b885109424 service_manager::SandboxLinux::InitializeSandbox()
#4 0x55b885107480 <unknown>
#5 0x55b887b17865 <unknown>
#6 0x55b887b1745f <unknown>
#7 0x55b8838a9b07 <unknown>
#8 0x55b8838aac8d <unknown>
#9 0x55b8838dc42b <unknown>
#10 0x55b8838a9111 <unknown>
#11 0x55b887c68488 headless::(anonymous namespace)::RunContentMain()
#12 0x55b887c6832e headless::RunChildProcessIfNeeded()
#13 0x55b8838d96b5 headless::HeadlessShellMain()
#14 0x55b8819f11ac ChromeMain
#15 0x7f60ab39c830 __libc_start_main
#16 0x55b8819f102a _start
Received signal 6
#0 0x55b883ccd7df base::debug::StackTrace::StackTrace()
#1 0x55b883ccd351 base::debug::(anonymous namespace)::StackDumpSignalHandler()
#2 0x7f60b1514390 <unknown>
#3 0x7f60ab3b1428 gsignal
#4 0x7f60ab3b302a abort
#5 0x55b883ccc185 base::debug::BreakDebugger()
#6 0x55b883c473e2 logging::LogMessage::~LogMessage()
#7 0x55b88510b223 sandbox::ProcUtil::HasOpenDirectory()
#8 0x55b885109424 service_manager::SandboxLinux::InitializeSandbox()
#9 0x55b885107480 service_manager::Sandbox::Initialize()
#10 0x55b887b17865 content::RendererMainPlatformDelegate::EnableSandbox()
#11 0x55b887b1745f [1106/144734.001287:FATAL:proc_util.cc(97)] Check failed: fstatat(proc_self_fd, de->d_name, &s, 0) == 0.
#0 0x55895e1b37df base::debug::StackTrace::StackTrace()
#1 0x55895e12cf70 logging::LogMessage::~LogMessage()
#2 0x55895f5f1223 sandbox::ProcUtil::HasOpenDirectory()
#3 0x55895f5ef424 service_manager::SandboxLinux::InitializeSandbox()
#4 0x55896168b955 content::(anonymous namespace)::ContentSandboxHelper::EnsureSandboxInitialized()
#5 0x55895f2c95ff gpu::GpuInit::InitializeAndStartSandbox()
#6 0x55896168b0d9 content::GpuMain()
#7 0x55895dd90c8d content::ContentMainRunnerImpl::Run()
#8 0x55895ddc242b service_manager::Main()
#9 0x55895dd8f111 content::ContentMain()
#10 0x55896214e488 headless::(anonymous namespace)::RunContentMain()
#11 0x55896214e32e headless::RunChildProcessIfNeeded()
#12 0x55895ddbf6b5 headless::HeadlessShellMain()
#13 0x55895bed71ac ChromeMain
#14 0x7f60d8a59830 __libc_start_main
#15 0x55895bed702a _start
which then seems to loop.
About this issue
- Original URL
- State: closed
- Created 6 years ago
- Reactions: 9
- Comments: 30 (7 by maintainers)
If anyone is still wondering about this: Im my case im not using puppeter, but through googling the error this page was a hit. (In my case im developing something based on the chromium source)
Anyway, im getting the same error while trying to run my chromium based executable through Windows WSL.
The code at fault is here: https://github.com/chromium/chromium/blob/master/sandbox/linux/services/proc_util.cc#L97
Its the gpu sandbox that is performing this check, to see if theres open dirs on the sandboxed process before starting the sandbox.
I´ve tried a simple code, almost the same as the code from chrome to test if its a generic thing, and it turns out that launching the executable by hand return 0 on ‘fstatat()’ as expected, so i added a code to print the error linux (inside WSL) is getting to see whats happening… My guess is that is something to do with some permissions the GPU process does not have.
Will follow with the real answer to anyone also getting this error. I hope it helps.
Just a note that I’m having a similar experience as @jukra:
circleci/ruby:2.5.1-node-browsers
:dumpio
attrue
, shows the same errors as @rbairwellJust upgraded and seeing the same error when running headless chrome in an alpine dockerized image. This happens before running puppeteer, so I’m assuming its something to do with chrome or one of the run flags I’m using.
puppeteer@v1.10.0 Chrome r599821 Chrome 72.0.3582.0
And
puppeteer@next Chrome r609904
I was also encountering weird errors on a page where we are using Chart.js to render charts. I was able to render the page if I ran to code locally, but when using Docker, got Timeout issues without clear indication on the error itself. If I tried to render google.com for example, it worked fine on both cases. After launching Puppeteer with
dumpio: true
I saw the same error @rbairwell is seeing.Thanks to tips on this thread, I downgraded to Puppeteer 1.9.0 and everything is working fine. I also tried using
puppeteer@next
but had the same issue with that one as well.My Dockerfile is almost the same as browserless Dockerfile, but with a few tweaks related to our application.
@aslushnikov
Here is the script, which reproduces this on Docker / Linux:
The key is something to do with GPU or WebGL. The wikipedia url works, the Mapbox GL one does not. macOS is not affected by this bug, it works well for me.
There is a timeout error, but it’s only pointing to the page.goto code.
I have run into this same regression. Host is dedicated server (no virtualization) Ubuntu 16.04. Docker guest is Ubuntu 16.04.
Deploying 1.10 put system CPU load to 200%. Interestingly not user, but system. Normally puppeteer raises the user CPU.
Reverting to 1.9 fixed this.
Graph of CPU usage while having 1.10 deployed: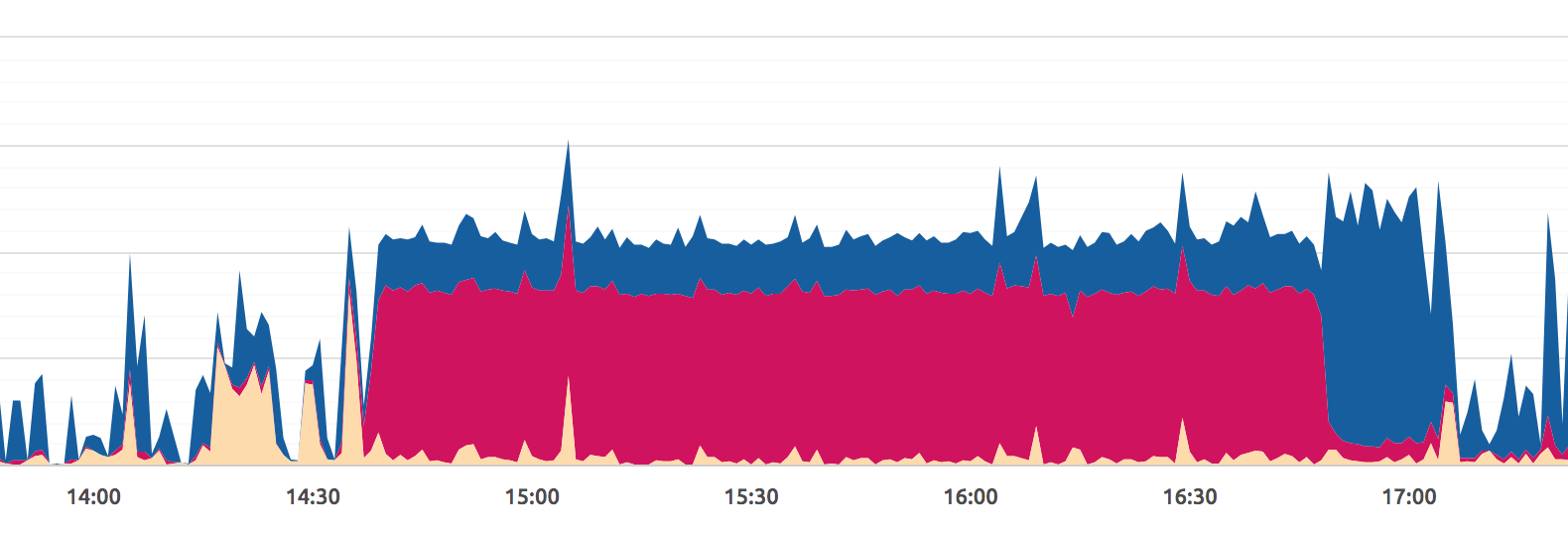
The blue bit at the end is the fixed 1.9 working on all the missed background tasks, and is how it should look.