puppeteer: [Bug]: Navigation timeout of 30000 ms exceeded with Puppeteer
Bug description
Firebase functions 3.18.0 Puppeteer 16.2.0 Node 16
Works fine in emulator, as soon as I deploy to the cloud it gives “Navigation timeout of 30000 ms exceeded” error 90% of the time just trying to launch the browser. I’ve even tried increasing the memory to 8GB and makes no difference.
const functions = require("firebase-functions");
const puppeteer = require("puppeteer");
exports.test = functions
.runWith ({memory: "512MB"})
.https
.onRequest(async (request, response) => {
var browser = await puppeteer.launch({
args: [ '--no-sandbox', '--disable-setuid-sandbox',],
headless: true,
});
try {
const page = await browser.newPage();
await page.goto("https://example.com");
// SEND RESPONSE
return response.send(JSON.stringify({
message : "success" }));
} catch (e) {
return response.send(
JSON.stringify({
error : true,
errorMessage : e.message
})
);
} finally {
await browser.close();
}
});
Puppeteer version
16.2.0
Node.js version
16
npm version
8.5.0
What operating system are you seeing the problem on?
macOS
Relevant log output
TimeoutError: Navigation timeout of 30000 ms exceeded
at LifecycleWatcher._LifecycleWatcher_createTimeoutPromise (/workspace/node_modules/puppeteer/lib/cjs/puppeteer/common/LifecycleWatcher.js:164:12)
at async Frame.goto (/workspace/node_modules/puppeteer/lib/cjs/puppeteer/common/Frame.js:169:21)
at async Page.goto (/workspace/node_modules/puppeteer/lib/cjs/puppeteer/common/Page.js:1144:16)
at async /workspace/index.js:73:9
About this issue
- Original URL
- State: closed
- Created 2 years ago
- Comments: 21
Increasing timeout makes no difference. Just fails after 1min rather than 30seconds
I’ve had a similar problem loading a script on my local machine and added added the timeout to 0 and wait until domcontentloaded:
that appears to work for me.
Update: I moved my Firebase Function from ‘us-central1’ region to ‘us-east4’ region… and now it works, same code. So the issue must have been with Firebase’s network in us-central1.
I am having the exact same experience. When it works, the response comes back in about 4 seconds (a reasonable amount of time for this particular page to load). when it does not come back, it fails after the 30 seconds. it is very random and I am currently always fetching the same page which is very basic. it either comes back in 4 seconds or never comes back, nothing in between. Mine is deployed on Dreamhost on a VPS the only difference in my code is that I am waiting on domcontentloaded vs just load.
here is the error: (masking my file system with “…”)
…node_modules/puppeteer/lib/cjs/puppeteer/common/LifecycleWatcher.js:164 return new Errors_js_1.TimeoutError(errorMessage); ^
TimeoutError: Navigation timeout of 30000 ms exceeded at LifecycleWatcher._LifecycleWatcher_createTimeoutPromise (…node_modules/puppeteer/lib/cjs/puppeteer/common/LifecycleWatcher.js:164:12)
Chromium version: HeadlessChrome/105.0.5173.0
±- puppeteer@16.2.0
I also tried to downgrade to 16.1.1 and had the same experience, which seems really odd.
This works more often than not, but doesn’t catch timeout and causes crashes… I would rather have a crash and more working sessions…
THIS SHOULD WORK! BUT 80% OF THE TIME IT IS CATCHING A TIMEOUT. IS IT FIREBASE ISSUE OR PUPPETEER? Why are so many sessions timing out?
It works on my local machine, but as soon as I deploy the function most of the requests timeout, but because some work I know the code is fine, so I’m really stuck on troubleshooting it. In the cloud timeout: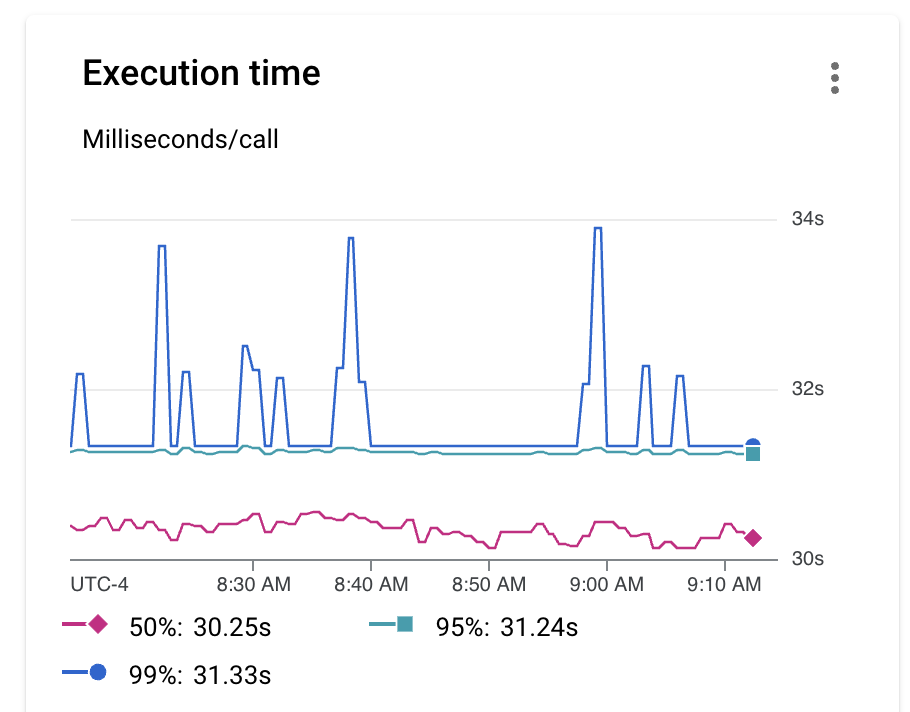
Here is the difference between using try/catch (left side of chart) and without (right side):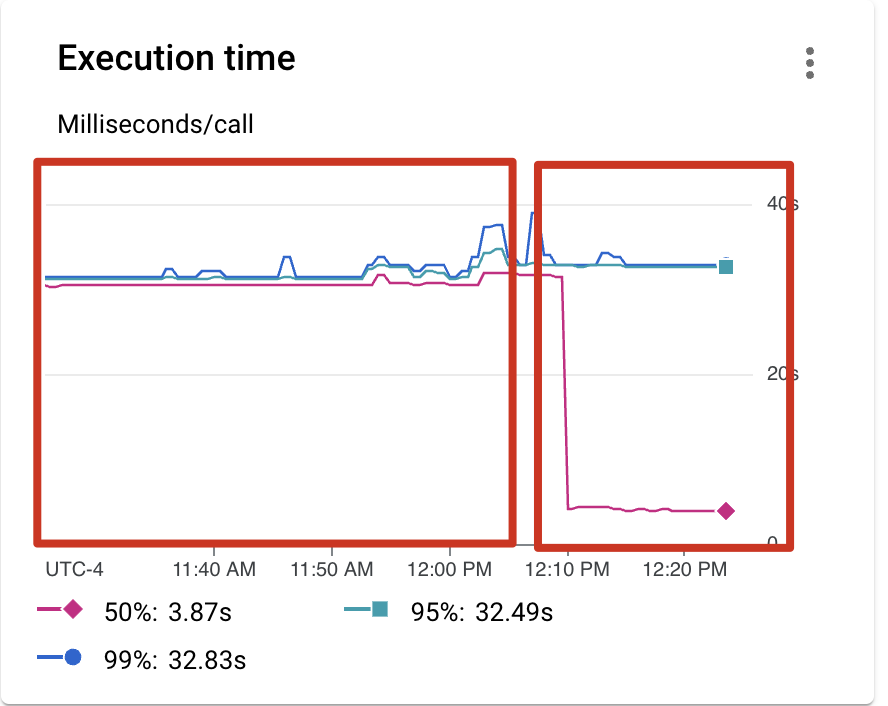