jest: Invalid hook call after `jest.resetModules` for dynamic `require`s
🐛 Bug Report
Invalid hook call when using jest.resetModules
or jest.resetModuleRegistry
with dynamic require
in test
To Reproduce
Steps to reproduce the behavior:
Expected behavior
No invalid hook call
Link to repl or repo (highly encouraged)
envinfo
System:
OS: macOS Mojave 10.14.6
CPU: (8) x64 Intel(R) Core(TM) i7-4850HQ CPU @ 2.30GHz
Binaries:
Node: 10.16.3 - ~/.nvm/versions/node/v10.16.3/bin/node
Yarn: 1.17.3 - /usr/local/bin/yarn
npm: 6.11.3 - ~/.nvm/versions/node/v10.16.3/bin/npm
npmPackages:
jest: 24.9.0 => 24.9.0
About this issue
- Original URL
- State: closed
- Created 5 years ago
- Reactions: 74
- Comments: 20
Commits related to this issue
- test(server): retry module tests These tests are flaky, as sometimes Jest chooses to cache the modules accross tests, even if they shouldn't be. isolatedModules or resetModules could help, but then r... — committed to algolia/react-instantsearch by Haroenv 2 years ago
- test(server): retry unit tests (#3475) * test(server): retry module tests These tests are flaky, as sometimes Jest chooses to cache the modules accross tests, even if they shouldn't be. isolatedMo... — committed to algolia/react-instantsearch by Haroenv 2 years ago
- test(server): retry unit tests (algolia/react-instantsearch#3475) * test(server): retry module tests These tests are flaky, as sometimes Jest chooses to cache the modules accross tests, even if th... — committed to algolia/instantsearch by Haroenv 2 years ago
I have also been running into this issue in a particularly nasty way. We use the
resetModules
config option globally, so tricks around callingjest.isolateModules()
don’t work well. Instead, I came up with a workaround that globally excludes React from jest’sresetModules
behavior.In a file specified in the
setupFiles
config option, add the following:This sets up a mock for React that lazily loads the actual React, and always returns the result of the first initialization. This could also be generalized to implement a set of modules that are globally excluded from
resetModules
.Generalized version:
Personally, I’ve found this to be a useful construct, and now that the React hook issue is more prevalent, it might be good to have a version of this built in to Jest as something like a
persistentModules
orexcludeFromResetModules
option.@kapral18 We are also experiencing the same issue. Have you found a work around for this?
The solution I came up with to our instance of this issue was more along the lines of solutions from Webpack and NPM: tell Jest to always use the same instance of React:
jest.config.json
:I had the same issue, instead of making any changes in setup files I end up with using https://jestjs.io/docs/en/jest-object.html#jestisolatemodulesfn in order to isolate each module so they are imported without having cache.
ex.
@lukevella Sorry for late response.
Here is what I came up with: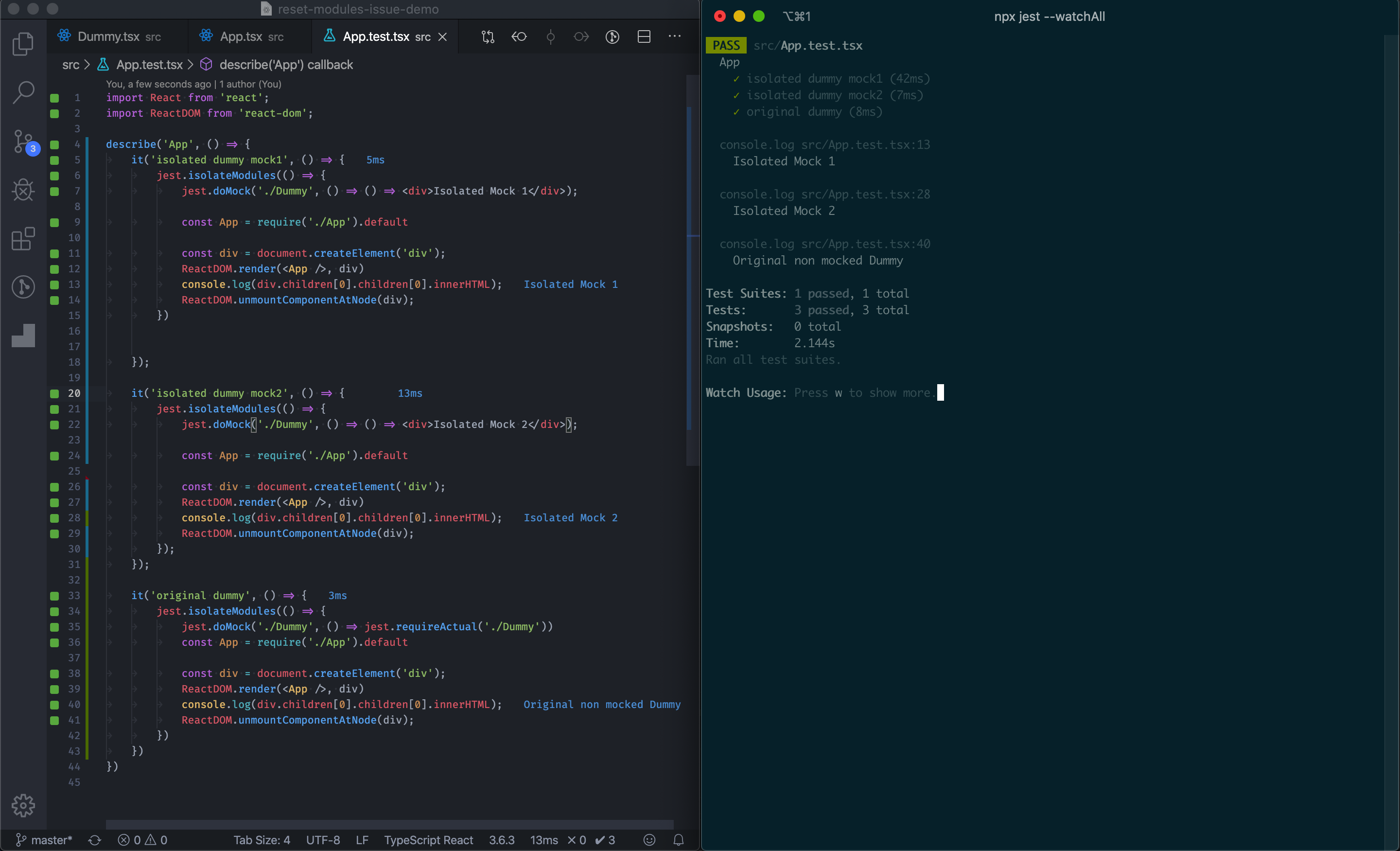
Seems like if you wrap each
jest.doMock()
call into ajest.isolateModules()
call in each test, - it actually re-mocks the modules, as opposed to basic behavior when the mocks are unchanged.And when you need to “reset” the mock, you
doMock
it again but withjest.requireActual
call.Thx @bwhitty,
require.resolve
also works for me.Thank you all so much. This was killing me all afternoon!
resetModules and isolateModules all caused issue for me. I just reinitialized the component to Test in the beforeEach state to get a fresh state:
same issue on my side.
I am also facing the same issues when I import react component(using hooks inside the component) using require and jest.resetModules() in the test.