fiber: 🔥 Docs: Error handling
Echo
I really like echos way of returning an error from your handler, even though I was sceptical at first. What I liked was:
- that it got rid of the case where an endpoint just returning nothing because I just logged the error and was to lazy to return an error message.
- forced to handle all error messages, even for
c.JSON
orc.Render
because I had to return something.
It’s understandable that you don’t want to break the handlers for backwards compatibility.
Fiber: Current State
Your concept of Next
and Error
does look interesting. But it is a bit difficult piecing together the necessary information (from the Docs, Examples and Github). And just from reading it, I still can’t fully understand it 🤷♂️
At the same time, there are various code snippets in the Docs or on GitHub were either log.Fatal is used or the error is silently swallowed.
Fiber: Improvements
It would be great to have a page in your docs about how YOU would implement error handling with fiber.
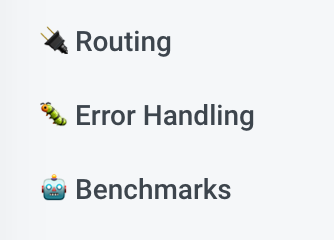
So how could I achieve something similar to echo? Here are the topics that I would like to have on such an “Error handling” page:
- return a JSON error message
{"messsage": "..."}
for/api
. - return an HTML error message for the views.
- return different status codes based on the error.
- how to recover from a panic.
- how to log the error and information about the request (to a different logger).
- if I call
Next
, in what order do the handlers execute. How to make sure that my error handler gets called?
These are just the things that come to mind right now. Take it with a grain of salt and maybe ask some other people what is important to them…
About this issue
- Original URL
- State: closed
- Created 4 years ago
- Comments: 15 (10 by maintainers)
@pharrisee, @hendratommy, @itsursujit, @JohannesKaufmann, thank you guys for participating in this discussion. With the feedback in this issue and on discord we have the following solution regarding error handling for
v1.11.x
https://docs.gofiber.io/error-handlingLet me know what you think of these changes so far, feedback is always welcome 👍
That looks interesting but maybe we should adopt the express error handling method and use an
ErrorHandler
that is triggered when callingc.Next(err)
I’m not sure that the recover mechanism should be in the error handler. Since the errorhandler can be overidden by developers that recover functionality would/could be lost?
A separate recover functionality would probably be better?
@Fenny I think the name
makes sense??