firebase-tools: Firestore data in emulator not showing up in emulator UI
[REQUIRED] Environment info
firebase-tools:
$ firebase --version
8.4.2
$ node -v
v10.16.3
Platform: macOS
[REQUIRED] Test case
index.js
const admin = require('firebase-admin');
process.env.FIRESTORE_EMULATOR_HOST = 'localhost:8080';
admin.initializeApp();
let db = admin.firestore();
async function test() {
let docRef = db.collection('users').doc('alovelace2');
let setAda = docRef.set({
first: 'Ada',
last: 'Lovelace',
born: 1815
});
}
test()
package.json
{
"name": "firebase-emulator-ui-test",
"version": "1.0.0",
"main": "index.js",
"author": "Chris Hager <chris@linuxuser.at>",
"license": "MIT",
"dependencies": {
"firebase-admin": "^8.12.1"
}
}
I simply created a new directory, initialise firebase with firebase init
and the node project with yarn init
, and added firebase-admin
(yarn add firebase-admin
).
[REQUIRED] Steps to reproduce
- Run the code above. Adding multiple entries to the firestore works, and I can retrieve them in code.
[REQUIRED] Expected behavior
Firebase UI at http://localhost:4000/firestore should show the data.
[REQUIRED] Actual behavior
Firebase UI at http://localhost:4000/firestore shows only an empty root.
About this issue
- Original URL
- State: closed
- Created 4 years ago
- Comments: 21 (3 by maintainers)
@charles-allen you’ve got it exactly right. A fake project ID will allow database writes but not much else. If you want to start the Emulator Suite with a fake project ID just do this:
Found the answer – when calling
admin.initializeApp()
you need to set the correctprojectId
, then the data will show up.Thanks! That was the missing piece of the puzzle.
Can I suggest (or even PR) to add the above to the docs here (where it mentions setting the fake project ID; since the snipper only shows the Admin SDK side, which doesn’t fully work without the above).
I actually Googled quickly, and I can’t find any reference to
--project
in the docs at all (only on other sites).To add to this, I got the same issue when creating different project environments via
firebase use [your environment name]
. The local emulator was working, just not showing any data. Once I switched my config settings to the correct environment, all was well and data started showing up in the emulator.@samtstern - In my case, I’m pretty sure that this error is the result of attempting to use a fake id (intentionally or accidentally). When I initialize with the same id as
firebase use
, everything works; when using a fake ID:Based on the docs and your comments, I’m not convinced it’s even possible.
From the docs you quoted:
However, over here you said:
To my knowledge, the current project is set using
firebase use
; when I attempt this with a fake id, I get this error:So how is it possible to set a fake id for firebase-tools, and thus for the emulator?
My script does set the projectId, and I still have this issue. I am running firebase 8.16.2.
My scripts are reading from and writing to the emulator fine, but if I go into the Emulator UI, there is no data shown. I can only view the collections, documents, subcollections, etc. if I manually create the top-level collection and documents. If I use the Cloud Firestore without the emulator, the same scripts work fine and I can view all the data in the UI without any manual intervention. Please advise.
@samtstern Well that is absolutely delightful for a person who knows where to find it. Google documentation together with Microsoft has always been a wall-of-text mess.
Embarrassing that this isn’t in the documentation more clearly.
I am using docker and have never gotten data to load, even with
admin.initilizeApp
using credentials for my existing project,firebase use my-project
etc. (also doesn’t work without docker and just usingfirebase --project project-id emulators:start
, no data in ui)how was this issue solved? I am still facing it
I had the same problem, using python library, and what worked for me was instantiating the firestore client with
db = firestore.client()
notdb = firestore.Client()
(Client capitalized). With instance and executingdb.project
returns different responses:client
=><my_project_id>
Client
=>google-cloud-firestore-emulator
@basickarl calling something embarrassing isn’t very constructive, also this IS in the documentation:
Link: https://firebase.google.com/docs/emulator-suite/connect_firestore#admin_sdks
Screenshot: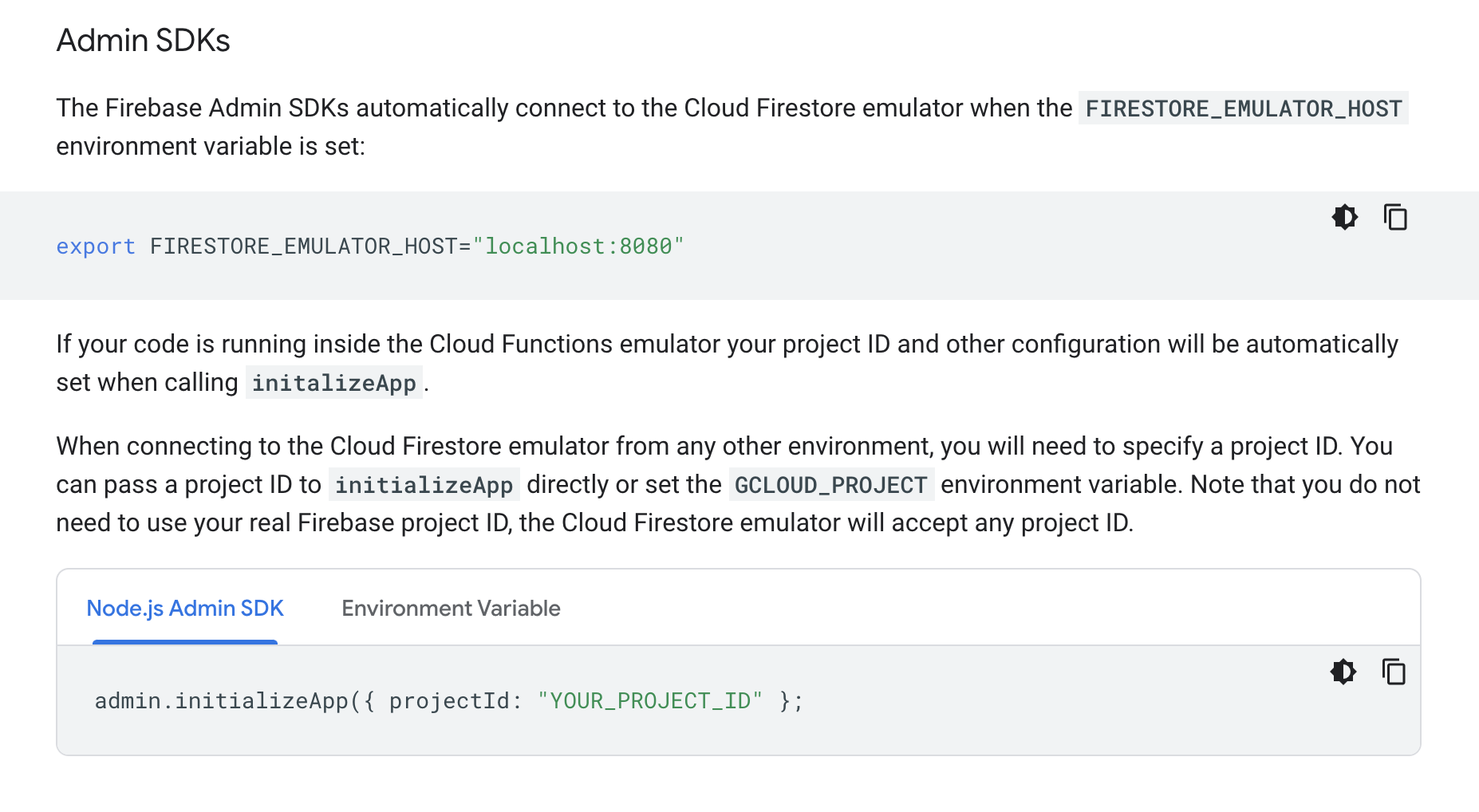
Adding my findings here. I was basically at the same place as this comment https://github.com/firebase/firebase-tools/issues/2365#issuecomment-749396838 and had resigned to use a real project id when running the emulator so that the active project in the CLI (
firebase use
) would matchadmin.initializeApp({ projectId: xx })
andfirebase --project project-id emulators:start
.However, as stated in the Emulator docs:
This felt really bad tbh, a loaded gun waiting to go off when someone thinks they’re working against an emulator and does a mistake that mutates real data.
However what the docs also says is interesting:
This was news to me. Turns out if you use the
demo-
prefix in your non-existing, fake project id, it won’t matter whatever is active in the CLI (firebase use
).The final state that got everything working for me:
npx firebase --project=demo-eaardal-emulator emulators:start"
npm run generate:local
):.env.local:
Data is showing in the Firestore Emulator UI both when using
--export-on-exit ./localdata --import ./localdata
and not.Just to verify, I have a real test project set as my active CLI project (
firebase use {real test project}
) and can verify that if I try to use a non-emulated service that exists and is active in the real test project, it will simply fail and not fall back on using the real project.Hope this helps!