firebase-functions: Cloud functions first call to Firestore very slow
We are experiencing extreme cold boot times, even with a small function like helloWorld, it takes like 5-6 seconds when firestore is used. Please see the following examples. The first function is done very fast, less than a second, I get the response “works”, and the second time, after querying firestore, takes 6 seconds: Function Version 1:
export const helloWorld = functions.https.onRequest(async (req, res) => {
console.log(process.env.FUNCTION_NAME + ' has started');
const admin = await import('firebase-admin');
admin.initializeApp();
res.status(200).json('works');
)}
Function Version 2, takes 6 seconds to return the user
export const helloWorld = functions.https.onRequest(async (req, res) => {
console.log(process.env.FUNCTION_NAME + ' has started');
const admin = await import('firebase-admin');
admin.initializeApp();
const userCol = await admin.firestore().collection('users').limit(1).get();
const userDoc = userCol.docs[0];
const user = userDoc.data();
res.status(200).json(user);
)}
I am using Node 8, and these dependencies: “firebase”: “^5.9.1”, “firebase-admin”: “^7.1.1”, “firebase-functions”: “^2.2.1”,
Related issues
About this issue
- Original URL
- State: closed
- Created 5 years ago
- Comments: 23 (8 by maintainers)
Reopen this issue! This is not solved!
For anyone who still has this problem, here’s my hack that help reduce initial firestore call from
~5s
to~80ms
.Reason is, google-admin sdk lazily loads gRPC Firestore and gRPC module. we need to get around that by making a random db query as following:
It might also be necessary and beneficial to run functions with
minInstances
NOTE: you will have to pay for these minInstances!
Good luck!
Why this issue is closed. This issue should be reopened
If this problem is affecting you, please upvote https://github.com/googleapis/google-cloud-node/issues/2942#issuecomment-604268183
reopen this issue
same problem happening with firebase functions
This issue is closed because there is nothing in the
firebase-functions
repo that can be done to solve it. The correct place to follow up is: https://github.com/googleapis/google-cloud-node/issues/2942@hitchingsh I have some good news - this will be the new default in the next functions release since we will be using the latest Firestore library that defaults to the smaller gRPC library. This release is coming very soon. For the meantime though, you can set that environment variable in the cloud console by going to Cloud Functions, selecting the function, and clicking Edit. Expand the list and at the bottom you’ll see “+ Add variable”: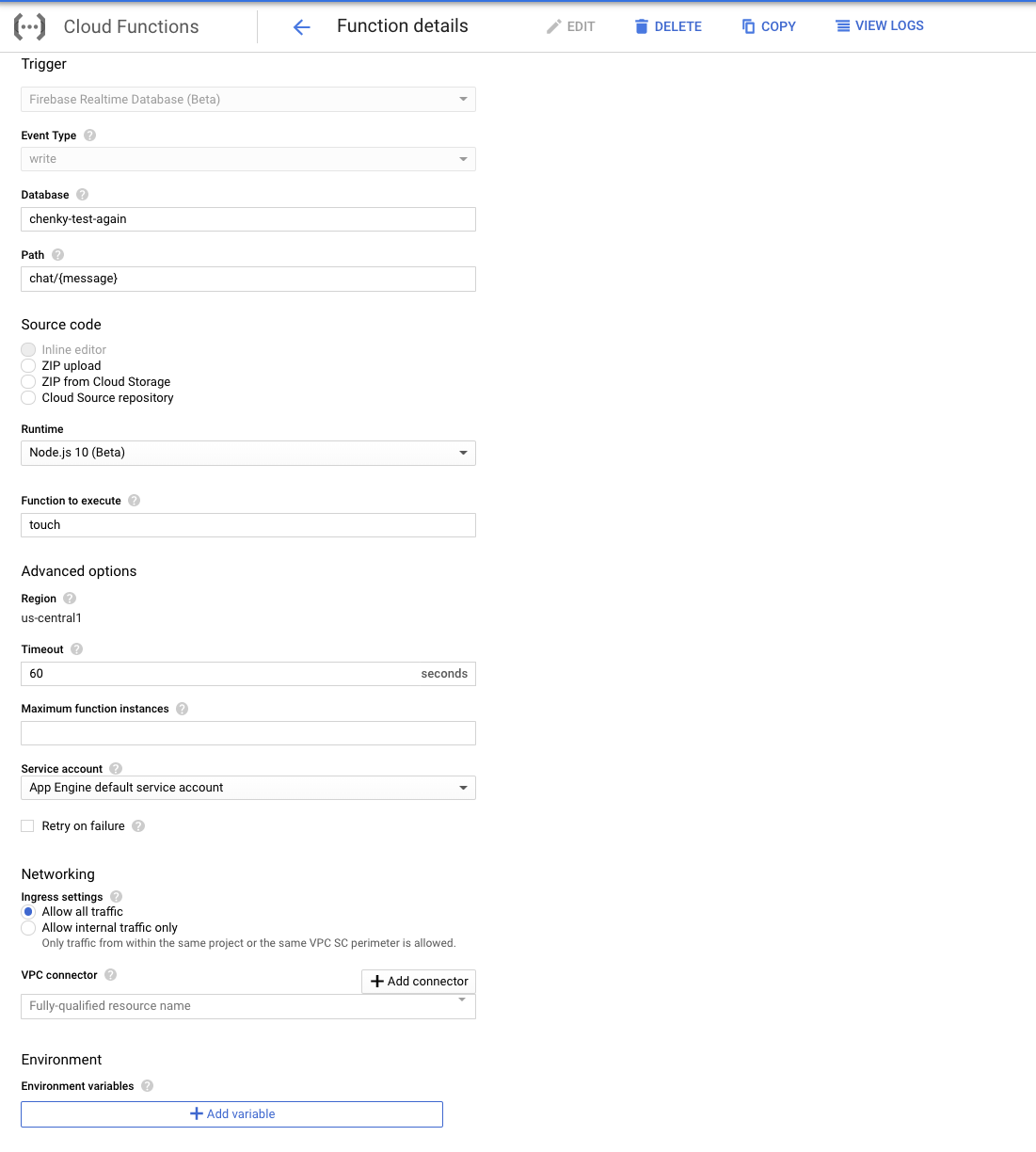
I think, it can help https://www.youtube.com/watch?v=v3eG9xpzNXM
Hi @xkLander thank you for filing this, I’m sorry to hear you’re having this issue. The first call to Firestore is quite heavyweight unfortunately since we have to create a gRPC connection under the hood and that takes some time. I’m curious - on subsequent runs of the V2 function, does it return faster? Since the connection to Firestore is established, it should be faster to call Firestore a second time the function is invoked (assuming it’s still on the same warm instance).