react-native: zIndex is broken across ScrollViews
π Bug Report
zIndex is broken when you have a ScrollView between your two different zIndexes. For example, given code like
render() {
return <View style={styles.background}>
<View style={styles.redBox}></View>
<View>
<View style={styles.greenBox}></View>
</View>
</View>
}
let styles = StyleSheet.create({
background: {
backgroundColor: "blue",
height: "100%"
},
greenBox: {
backgroundColor: "green",
width: 100,
height: 100,
zIndex: 2
},
redBox: {
height: 300,
width: 300,
backgroundColor: "red",
position: "absolute",
top: 0,
left: 0,
zIndex: 1
},
});
you get a view that makes sense, blue background, then red box, then green box on top.
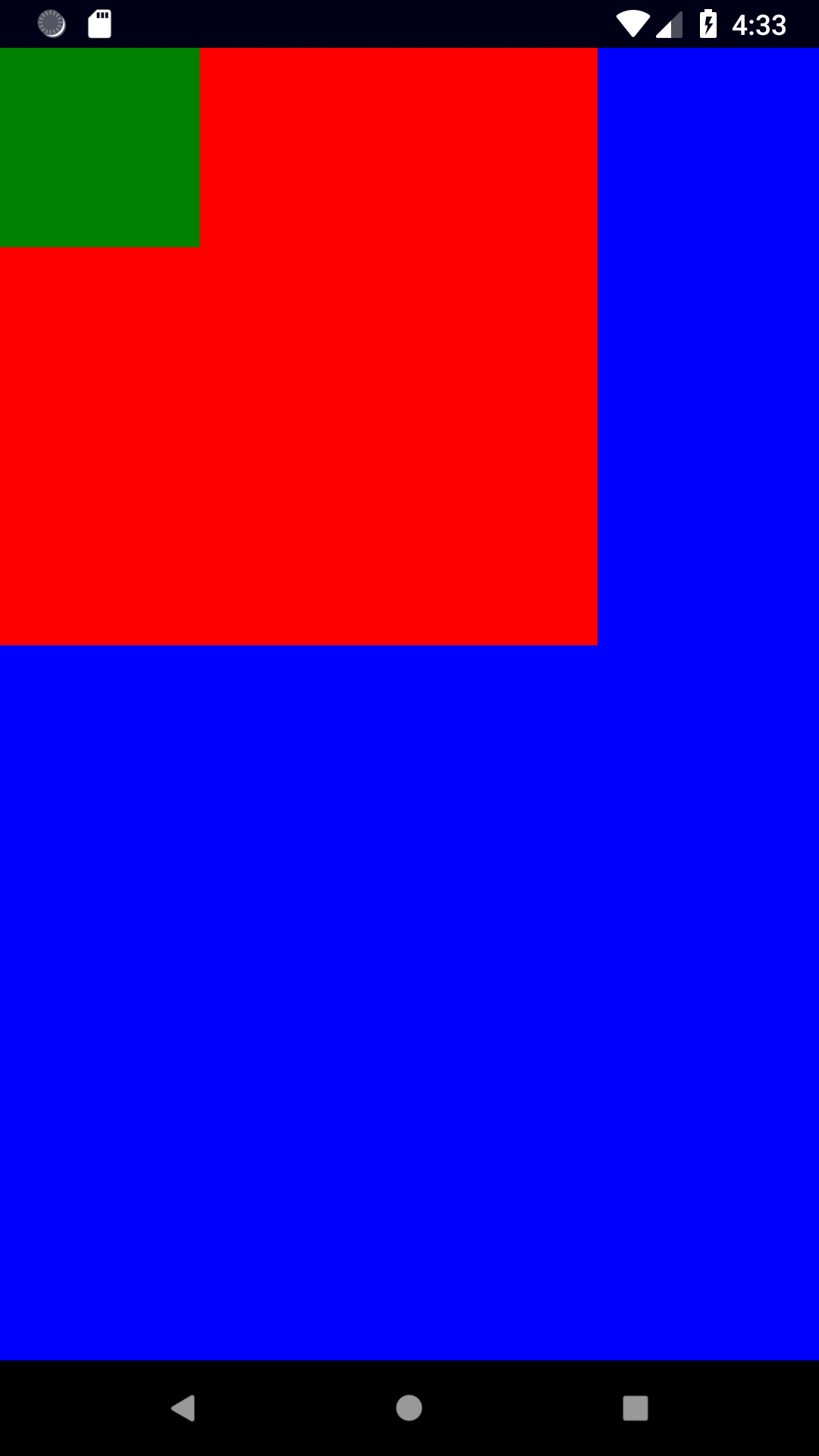
However, if you just switch the container of the green box to a ScrollView, the zIndex breaks:
<View style={styles.background}>
<View style={styles.redBox}></View>
<ScrollView>
<View style={styles.greenBox}></View>
</ScrollView>
</View>
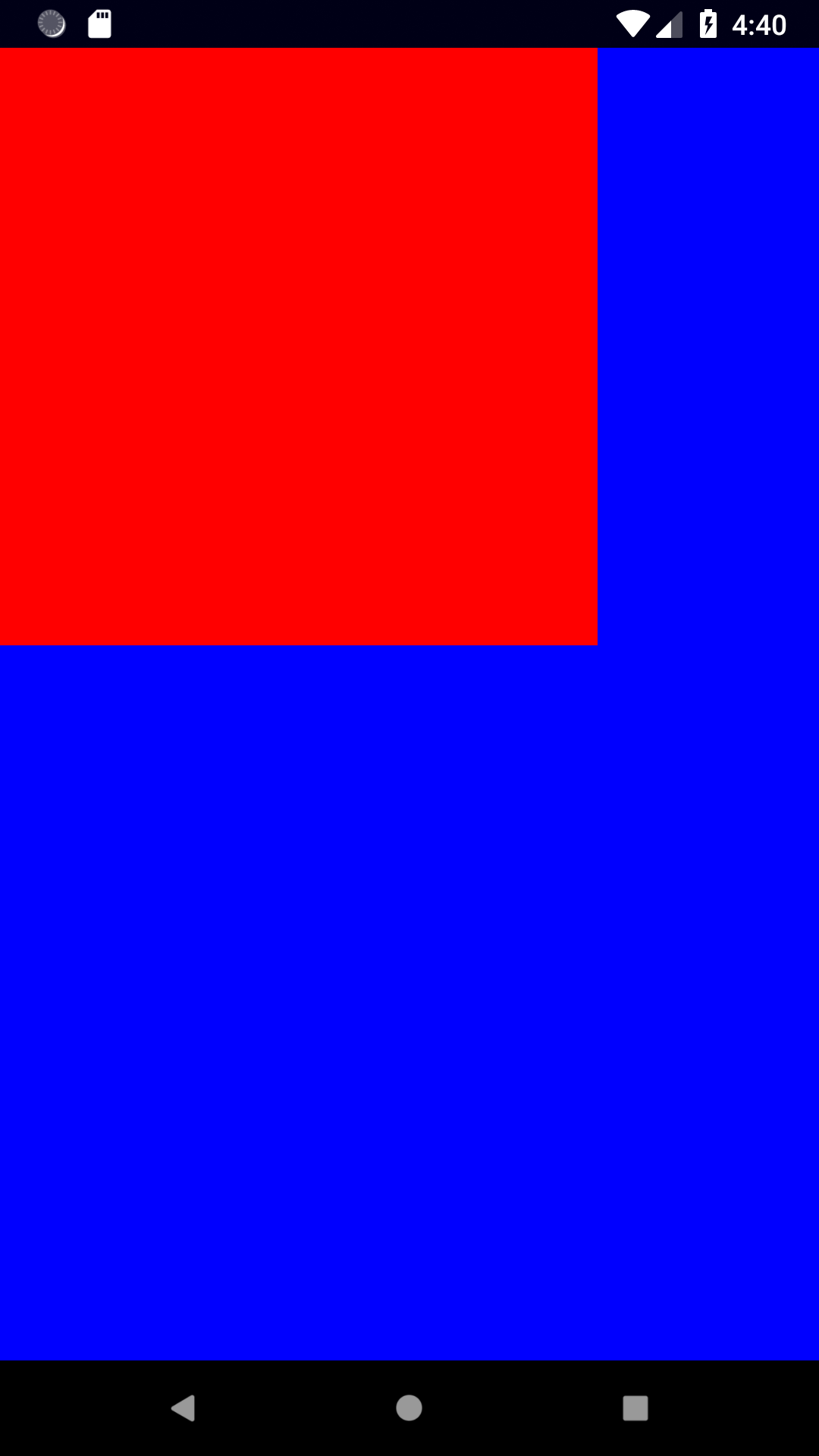
Expected Behavior
Components inside of a ScrollView should still respect zIndex.
Code Example
import React, { Component } from 'react';
import {
View,
ScrollView,
StyleSheet,
} from 'react-native';
export default class App extends Component {
render() {
return (
<View style={styles.background}>
<View style={styles.redBox}></View>
<ScrollView>
<View style={styles.greenBox}></View>
</ScrollView>
</View>
);
}
}
let styles = StyleSheet.create({
background: {
backgroundColor: "blue",
height: "100%"
},
greenBox: {
backgroundColor: "green",
width: 100,
height: 100,
zIndex: 2
},
redBox: {
height: 300,
width: 300,
backgroundColor: "red",
position: "absolute",
top: 0,
left: 0,
zIndex: 1
},
});
Environment
Environment: OS: Windows 10 Node: 9.3.0 Yarn: 1.3.2 npm: 4.6.1 Watchman: Not Found Xcode: N/A Android Studio: Version 3.2.0.0 AI-181.5540.7.32.5056338
Packages: (wanted => installed) react: 16.3.2 => 16.3.2 react-native: ^0.55.4 => 0.55.4
Itβs worth noting that on iOS not even the working example I give here works, it doesnβt show the green box above the red one ever.
About this issue
- Original URL
- State: closed
- Created 5 years ago
- Reactions: 3
- Comments: 22 (1 by maintainers)
RN 0.62.2 also having this issue , Any solution for this issue?
@fabriziobertoglio1987 Hey, you tested it in only one scrollview. I mean drag/drop between two scrollviews.
I am unable to completely understand what you said, but I think you can have different flex values for both scroll views. But I doubt zIndex works as expected with siblings of different parents. Please try it out and let us know. I also will be prepare a better implementation of my functionality if that works.
Scrollview with zIndex, and position absolute works someitmes and fails sometimes, still facing this issue.
can you close? I tested on android
https://snack.expo.io/@fabrizio.bertoglio/supportive-graham-crackers
for me it works in in scrollView i provide in contentContainerStyle={{flex:1}}.