react-native: Switch does not save state when swipe gesture is used
Is this a bug report?
Yes
Have you read the Contributing Guidelines?
Yes
Then, specify:
- Target Platform: 22
- Development Operating System: Windows
- Build tools: “23.0.1”
(edits by @hramos) Environment
react-native -v:
node -v:
npm -v:
yarn --version:
Steps to Reproduce
(Write your steps here:)
- Create wrapper component for Switch
//@flow
import React, { PureComponent } from "react";
import { Switch } from "react-native";
import PropTypes from "prop-types";
export default class Switcher extends PureComponent {
static propTypes = {
value: PropTypes.bool.isRequired,
onToggle: PropTypes.func.isRequired
};
render() {
const { onToggle, value } = this.props;
return (
<Switch
onValueChange={onToggle}
value={value}
tintColor="#dadada"
thumbTintColor={value ? "#2287e1" : "#ffffff"}
onTintColor="#a7cff3"
/>
);
}
}
- Create component
class MyData extends PureComponent {
constructor(props) {
super(props);
this.state = { switchValue: false };
}
toggleSwitch = value => {
this.setState({ switchValue: value });
console.log(`Switch 1 is: ${value}`);
};
...
render(){
...
<Switcher
value={this.state.switchValue}
onToggle={this.toggleSwitch}
/>
...}
}
- Run project
- Make as shown in gif
Expected Behavior
Save start state into Switch
(Write what you thought would happen.)
Actual Behavior
Crash when pulling
Reproducible Demo
(Paste the link to an example project and exact instructions to reproduce the issue.)
About this issue
- Original URL
- State: closed
- Created 7 years ago
- Comments: 21 (4 by maintainers)
Is this still an Issue ? If so, Can I pick this up ?
Hi. I want to clarify a problem. The component expecting to change flag (true -> false or false -> true), but if you save position of point after move from the edge to the edge this reproduce problem.
Start with flag “true”: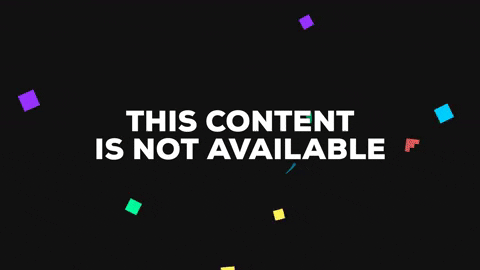
Start with flag “false”: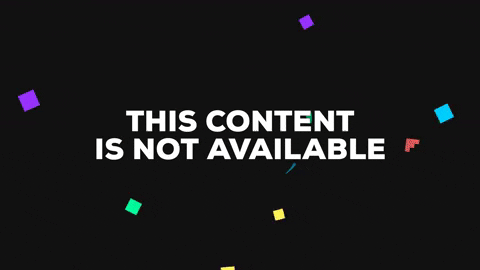
The surefire way to reproduce this bug is to start dragging, and then return to the state you started with (by letting go at some point before the half way point). The bug occurs when started from both true and false states.
That being said, I’m still seeing it on Android with RN-0.48.0
@zibs I’m able to recreate on that snack using Expo on my google pixel. You might need to drag around a few times and make sure you really get that quick drag “swipe” action in there.
Easy way to recreate is to drag to the left when the switch is already in the off state.
FWIW I already researched this issue and debugged it (see above) and know it is a problem.
@KirillTarasenko I’ve made a snack for you here: https://snack.expo.io/r1mGumMt-
It works, and I’ve just copied and pasted your code. I’m not sure if you can provide any other details for your issue? Maybe double-check your code and make sure it’s not something else you’ve misspelt or something.
Hey, thanks for reporting this issue!
It looks like your description is missing some necessary information, specifically I do not see any mention of the React Native version you are using. Can you please add all the details specified in the template? This is necessary for people to be able to understand and reproduce the issue being reported.