react-native: HTTP Fetch fails with "TypeError: Network request failed" => Resolved
Description
There are 83 issues opened and unanswered about network requests failing with this generic error. The main causes of the pain are:
- Not getting an answer from the team
- The exception is far too generic and does not suggest the origin of the problem
Problem description
Using fetch
to get/post on a HTTPS web server which is using a valid and trusted but not public CA.
- Using Chrome and other apps the requests are always successful and without certificate problems
Sample code in react native:
static async Post(): Promise<string> {
let srv = "my.domain.com";
let port = 5101;
let device = "abcd";
let url = `https://${srv}:${port}/Do/Something?devicename=${device}`;
try {
let response = await fetch(url, {
method: 'POST',
headers: {
'Accept': 'application/json',
'Content-type':'application/json'
},
body: JSON.stringify({
key: 'value',
})
});
if(response.status !== 200) throw new Error(`Can't open ${srv} for ${device} with status ${response.status}`);
return response.json();
}
catch(e) {
console.log(e);
throw(e);
}
}
Solution
Due to Android restrictions, a network_security_config
configuration must be added to the application. It is an xml file that can be added by following these steps:
- Edit the
android/app/src/main/AndroidManifest.xml
- Add the
android:networkSecurityConfig="@xml/network_security_config"
to the<application />
tag - Create the folder
android/app/src/main/res/xml
and inside a file callednetwork_security_config.xml
- If you don’t want to install the CA in the Android certificates, add the folder
android/app/src/main/res/raw
Variant 1: using the certificates added manually to Android.
In this case the CA must be visible in the User Certificates in the Android Settings. Try using them by opening a website that uses those certificates in Chrome to verify they are valid and correctly installed.
Content of the network_security_config.xml
<?xml version="1.0" encoding="utf-8"?>
<network-security-config>
<domain-config>
<!-- Localhost config is NEEDED from react-native for the bundling to work -->
<domain-config cleartextTrafficPermitted="true">
<domain includeSubdomains="true">127.0.0.1</domain>
<domain includeSubdomains="true">10.0.0.1</domain>
<domain includeSubdomains="true">localhost</domain>
</domain-config>
<domain includeSubdomains="true">my.domain.com</domain>
<trust-anchors>
<certificates src="user"/>
<certificates src="system"/>
</trust-anchors>
</domain-config>
</network-security-config>
The <certificates src="user"/>
is the one giving access to the certificates installed manually.
Variant 2: using a certificate bundled with the app
You should export (using ssl) a pem certificate containing just the public key, naming it “ca” (no extension). Copy the certificate in the raw
folder
<?xml version="1.0" encoding="utf-8"?>
<network-security-config>
<domain-config>
<!-- Localhost config is NEEDED from react-native for the bundling to work -->
<domain-config cleartextTrafficPermitted="true">
<domain includeSubdomains="true">127.0.0.1</domain>
<domain includeSubdomains="true">10.0.0.1</domain>
<domain includeSubdomains="true">localhost</domain>
</domain-config>
<domain includeSubdomains="true">my.domain.com</domain>
<trust-anchors>
<certificates src="@raw/ca"/>
<certificates src="system"/>
</trust-anchors>
</domain-config>
</network-security-config>
Important note (added on June 22, 2022)
The local traffic (with the packager) must be unencrypted. For this reason the <domain-config />
must contain the clearTrafficPermitted=true
.
It is also important adding the ip addresses used from react-native when debugging otherwise the application will crash because of the android:networkSecurityConfig="@xml/network_security_config"
attribute. If you see the app crashing, take not of the ip used internally from react native and add it/them to this list. For example:
<domain-config cleartextTrafficPermitted="true">
<domain includeSubdomains="true">127.0.0.1</domain>
<domain includeSubdomains="true">10.0.0.1</domain>
<domain includeSubdomains="true">10.0.1.1</domain>
<domain includeSubdomains="true">10.0.2.2</domain>
<domain includeSubdomains="true">localhost</domain>
</domain-config>
Requested fix: please never throw exceptions with a generic message, they are only a huge pain.
Version
0.67.0
Output of npx react-native info
info Fetching system and libraries information… System: OS: Windows 10 10.0.19044 CPU: (8) x64 Intel® Core™ i7-6700 CPU @ 3.40GHz Memory: 8.20 GB / 31.93 GB Binaries: Node: 16.13.0 - C:\Program Files\nodejs\node.EXE Yarn: 1.22.4 - C:\Program Files (x86)\Yarn\bin\yarn.CMD npm: 8.1.3 - C:\Program Files\nodejs\npm.CMD Watchman: Not Found SDKs: Android SDK: API Levels: 19, 23, 25, 26, 27, 28, 29, 30 Build Tools: 19.1.0, 21.1.2, 22.0.1, 23.0.1, 23.0.3, 26.0.2, 27.0.0, 28.0.0, 28.0.3, 29.0.2, 30.0.2 System Images: android-27 | Google APIs Intel x86 Atom, android-27 | Google Play Intel x86 Atom Android NDK: 22.1.7171670 Windows SDK: AllowDevelopmentWithoutDevLicense: Enabled AllowAllTrustedApps: Enabled Versions: 10.0.10586.0, 10.0.14393.0, 10.0.15063.0, 10.0.16299.0, 10.0.17134.0, 10.0.17763.0, 10.0.18362.0, 10.0.19041.0 IDEs: Android Studio: Version 2020.3.0.0 AI-203.7717.56.2031.7935034 Visual Studio: 17.1.32104.313 (Visual Studio Enterprise 2022), 16.11.32002.261 (Visual Studio Enterprise 2019) Languages: Java: 1.8.0_302 npmPackages: @react-native-community/cli: Not Found react: 17.0.2 => 17.0.2 react-native: 0.66.4 => 0.66.4 react-native-windows: Not Found npmGlobalPackages: react-native: Not Found
Steps to reproduce
Use the above code to make an HTTPS request to a website protected with certificates that are not public. They will not succeed with a generic exception (as for the issue title)
Repeat the request to a public website and it will succeed. The issue is the exception being too generic.
Snack, code example, screenshot, or link to a repository
No response
About this issue
- Original URL
- State: open
- Created 2 years ago
- Reactions: 65
- Comments: 49 (1 by maintainers)
It is an habit in this repo to ignore important issues (not only this one) for a very long time. I start believing RN is not that worthy to invest in.
2023 and they haven’t addressed this generic exception issue. I’ve been stuck on this for days, trying every solution and nothing works. I just wanted to know what the actual error is instead of shooting on the dark.
My issue was fixed when I restarted the emulator
It’s simply sad that this catastrophic DX failure in RN has been around essentially since the beginning, yet there still is no clear roadmap for fixing it (or simply propagating the underlying errors to make it actually debuggable). It’s barely even being acknowledged, yet is one of the most asked-about problems with RN.
Thanks a ton @raffaeler for doing God’s work documenting issues like this, RN would more or less be dead without community members like you.
what do yo suggest for people using Expo, as there is no direct android folder of manifest file.
Still to this day, the error messages for fetch network errors are super super vague. Is this going to get fixed?
It might be worth noting that I saw this error when I attempted to send a
JSON.stringify'd
body, without the'Content-type':'application/json'
header.It looks like the underlying okhttp library has quite good error detection and reporting. Somehow though, either the react-native networking layer, or the whatwg-fetch doesn’t capture that!
Thank you @hoaxvo16 but I already posted the solution in this thread. My request is react-native providing a decent error so that nobody else has to spend hours in trying to resolve it. Also, since this an OSS project, it would be nice for some of the owner to “say something” instead of infinite silence.
POSIBLE SOLUTION
I faced the same problem (iOS working & Android failure) and in the end it turned out to be a problem because the domain to which the request was made contained an underscore (_) character. Removing the underscore by a dash solved the problem.
Previos domain https://my_domain.com
Working domain https://my-domain.com/
If you are using a domain other than localhost and use special characters it is possible that it may fail because of this. I will update my comment if I find any additional information.
I hope it helps 🙏🏻
Thank you for the reply. I have found the reason of that is because the manifest merger failed to merge
debug/AndroidManifest.xml
andmain/AndroidManifest.xml
, I make two AndroidManifest.xml have same user-permission configuration and it’s work right now.Lemme just jump in that I’m always an advocate for bubbling as much error info up the stack as possible. There are those of us who go to great lengths, perhaps even cursed lengths, to make use of the info that is available from web
fetch
. It does actually make a big difference in debugging for those of us who go to these lengths, it’s saved me untold hours of debugging, and its vastly more useful than the standard “failed to fetch” network error. If I had the time, and lacked the extreme disdain for Java, I’d write the patch myself!We were also facing similar issue in Production and multiple customers complained about the problem of not able to access our App due to this error. Upon extensive debugging, we found that our cloud infra only accepted requests which had IPv4 addresses. If any device network wasn’t able to allocate an IPv4 address then all such requests were getting dropped with the App receiving the “Network Request Failed” error. Hope this helps!
For the entertainment of other readers, here’s two issues I’ve opened about goofy issues I encounter when errors are not super well reported (or reportable) https://github.com/puma/puma/issues/2894 https://github.com/nodejs/node/issues/43439
This does not make any sense. The point of this entire thread is to use a non public CA as stated in the initial post, which is extremly common in any enterprise scenario.
The link you posted is a service that can only verify public websites. Also, I strongly reccomend to verify TLS (SSL does not exist anymore) using openssl utilities which are the most updated.
@MuhammadAbdullah54321 this issue is strictly related to fetching with HTTPS where the server is using a private or self-signed certificate. In this case, if you carefully follow my points you should resolve the issue.
It is very bad that meta is not even answering or even checking the issues. This should be clarified and be part of the official documentation.
I’ll try to find a solution for this specific problem elsewhere. Thank you for your support 🙏
Here’s my API (checking on Desktop Chrome):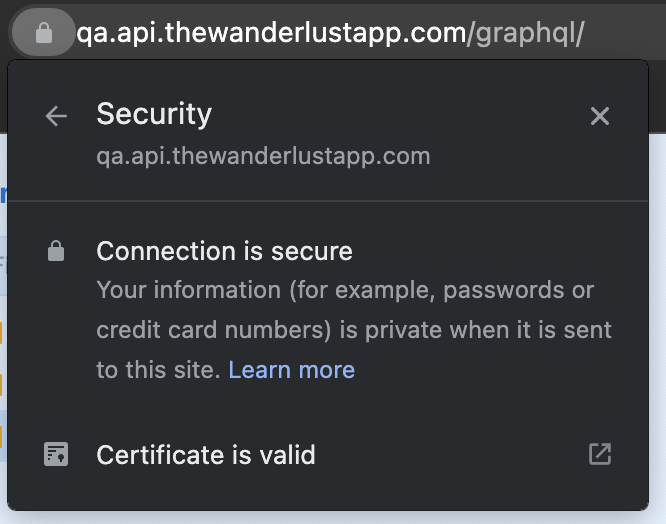
I am assuming it is publicly verifiable.
However, on those Android versions (from API 22 to API 24), I have the “Network request failed” error.
I noticed that trying to directly open the website on Chrome on those old Android versions brings this error, it should be related:
Certificate viewer:
So yeah, something weird on old Android version validating certificates?!
I have this problem connecting with my hosted API (with a valid SSL certificate from Let’s Encrypt) on Android Level 21 (Android 5), 22 (Android 5.1), 23 (Android 6) and 24 (Android 7).
After trying both variant 1 and 2 without success, I gave up and removed support for these Android versions (represent like 5% of market share to this day).
No idea what’s happening, the error doesn’t help 😞
@raffaeler yeah i know, took me over 3 hours to solve this, good thing is you learned something new 😄