babel-loader: .babelrc not working
I have folled a very simple tutorial to set up webpack, babel and react, but get an error with the presets when I use a .babelrc
file.
webpack.config.js:
var path = require('path');
module.exports = {
entry: './src/index.js', // Bundle all assets from this location together
output: {
filename: 'bundle.js', // Output to this file
path: path.resolve( __dirname, 'dist' ) // In this location
},
module: {
rules: [
{
test: /\.(js|jsx|mjs)$/,
loader: 'babel-loader',
exclude: /node_modules/,
},
{
test: /\.css$/,
use: [ 'style-loader', 'css-loader' ]
}
]
},
devServer: {
contentBase: path.resolve(__dirname, "dist")
}
};
.babelrc:
{
"presets": ["env", "react"]
}
Gets me the following error:
ERROR in ./src/index.js
Module build failed: SyntaxError: Unexpected token (17:12)
15 | render() {
16 | return (
> 17 | <div style={ { textAlign: 'center' } }>
| ^
If I change my webpack.config.js file to this instead it works:
var path = require('path');
module.exports = {
entry: './src/index.js', // Bundle all assets from this location together
output: {
filename: 'bundle.js', // Output to this file
path: path.resolve( __dirname, 'dist' ) // In this location
},
module: {
rules: [
{
test: /\.(js|jsx|mjs)$/,
exclude: /node_modules/,
use: {
loader: 'babel-loader',
options: {
presets: ['env', 'react']
}
}
},
{
test: /\.css$/,
use: [ 'style-loader', 'css-loader' ]
}
]
},
devServer: {
contentBase: path.resolve(__dirname, "dist")
}
};
From my understanding having the options object or a .babelrc file should do the same thing. But only one of them works.
I don’t have a "babel": {}
block within my package.json so I really don’t see why it seems that the .babelrc file is not recognized.
About this issue
- Original URL
- State: closed
- Created 7 years ago
- Reactions: 21
- Comments: 36 (7 by maintainers)
Commits related to this issue
- (test): add initial test harness - add 1 simple test for existence of canvas and instance methods - change test script to use jest, and move prev test to test:pub - change CI to run test and test:... — committed to agilgur5/react-signature-canvas by agilgur5 5 years ago
- (test): add initial test harness - add 1 simple test for existence of canvas and instance methods - change test script to use jest, and move prev test to test:pub - change CI to run test and test:... — committed to agilgur5/react-signature-canvas by agilgur5 5 years ago
- (test): add initial test harness - add 1 simple test for existence of canvas and instance methods - (pkg): test code shouldn't be in the package, just source - currently preferring to keep sour... — committed to agilgur5/react-signature-canvas by agilgur5 5 years ago
- (test): add initial test harness - add 1 simple test for existence of canvas and instance methods - (pkg): test code shouldn't be in the package, just source - currently preferring to keep sour... — committed to agilgur5/react-signature-canvas by agilgur5 5 years ago
- (test): add initial test harness - add 1 simple test for existence of canvas and instance methods - (pkg): test code shouldn't be in the package, just source - currently preferring to keep sour... — committed to agilgur5/react-signature-canvas by agilgur5 5 years ago
- (test): add initial test harness and one simple test - ensure that width/height of canvas are trimmed down after drawing a purple rectangle in the center - add test script that uses jest - (ci):... — committed to agilgur5/trim-canvas by agilgur5 5 years ago
- (test): add initial test harness and one simple test - ensure that width/height of canvas are trimmed down after drawing a purple rectangle in the center - add test script that uses jest - (ci):... — committed to agilgur5/trim-canvas by agilgur5 5 years ago
- (test): add initial test harness and one simple test - ensure that width/height of canvas are trimmed down after drawing a purple rectangle in the center - add test script that uses jest - (ci):... — committed to agilgur5/trim-canvas by agilgur5 5 years ago
- (test): add initial test harness and one simple test - ensure that width/height of canvas are trimmed down after drawing a purple rectangle in the center - add test script that uses jest - (ci):... — committed to agilgur5/trim-canvas by agilgur5 5 years ago
I have this code in my .babelrc and its working for me.
For more, I have my
and
postcss.config.js
which isI would like to tell you that I am using React with Semantic-ui-react and this configuration is rocking. You can try. If you face any error tell me.
From spelunking the same code, it appears that
options: { babelrc: true }
will also trigger the default behavior. I have no idea why that would not be the default, but hey.I was successfully using @finom’s solution above.
After upgrading to the official Babel 7 release, due to other issues, I eventually had to migrate
.babelrc
tobabel.config.js
by following these docs: https://babeljs.io/docs/en/next/configuration.html, which basically explain that anyone who needs to compile anything innode_modules
needs to usebabel.config.js
.Then I was able to remove that
JSON.parse...
hack, and everything seems to work great now!Direct read of .babelrc is helped for me:
I see the real issue now after some more investigation,
babel.transform
does not read.babelrc
file; however, all the other functions do such asbabel.transformFile|transformFileSync|...
. This loader is executingbabel.transform
, so you have to pass in the options. I’m not sure if this fix should be in the babel-loader project or the babel-core project.@al-the-x just to point out that
babelrc: true
is incorrect. If you take a look here, it’s actually a string type not a boolean type. And the string is supposed to be the relative path to the.babelrc
file.So the issue can be resolved in two ways:
babel-core#transform
to read the.babelrc
configurations oroptions.babelrc
it reads and parses it and passes it as theoptions
tobabel.transform
. Because nowhere in the code actually reads the.babelrc
file and passes it through.The babelrc path gets assign here, and then gets removed here, and transpile doesn’t get it. Where it needs to get added separately is here.
@skukan159
const fs = require('fs')
;None of the above solutions worked for me, but this is:
So actually, I’m getting the .babelrc file and use it’s contents as the options for babel-loader
Setting
babelrc
to a string results in the following for me:Module build failed: Error: .babelrc must be a boolean, or undefined
In my case using
babelrc: true
did what I was expecting; using the project’s.babelrc
file. Imo it would be nice if the.babelrc
file is used by default if it’s available.I encountered this issue on a fresh npm install of all packages when one of the folders involved had it’s own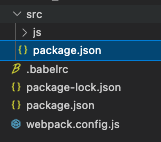
package.json
.Can you please test again with 8.0.0-beta.1. It should be fixed now.
https://github.com/babel/babel-loader/releases/tag/v8.0.0-beta.1
@mohsenuss91 Posting the same question in a lot of closed issues won’t get you an answer if you don’t provide more info.
Please, share your current configuration, your files/directories structure, the behavior you are seeing and the behavior you are expecting.
@pietrofxq
babel.config.js
is loaded from your working directory by default, so it would depend on what the working directory is.@al-the-x I readed the babel-laoder’s code, if babelrc is not false, it will find the .babelrc file from the executing directory, which not like node. But if the babelrc is string, like: babelrc: path.resolve(__dirname + “/.babelrc”), it works. But there is another thing, env is not founded, because the presets env is also be read from the excuting directory, it makes me crazy! So, I think we should not use the .babelrc file but config the babel options in the webpack.config.js file!
That’s not how I read that code, @nguyenj:
Refactored for more clarity using some lodash operations not in the original:
This explains the positive experience (boolean pun!) that @thasmo and I had:
Nice bit of detective work tracing the
babel.transpile
call, though. Since we know thatbabelrc:true
would work by default without a change to the upstreambabel
or using one of thetranspileFile|transpileFileSync
methods, perhaps we could makebabelrc
default totrue
in the options?The shorthand
babel-loader?babelrc
works for me, as well and is less to (remember to) type, but I’d still prefer the default.@nguyenj I think a better approach might be to highlight in the documentation that you have to set
babelrc:true
on the loader if you have a.babelrc
file in the project and to the path of the file if it’s not the default name and location. I’d prefer to see that option default totrue
, personally, as long as Babel doesn’t blow up if the file is missing.