axios: AxiosRequestHeaders type breaks from 0.21.4 to 0.22.0
Describe the bug
The type has changed and now I am getting linting errors on a working code.
I get Element implicitly has an ‘any’ type because index expression is not of type ‘number’.ts(7015) error when I try to access the headers by their name on a AxiosRequestHeaders
object.
For example accessing a common header in transfomRequest
where the content of headers is:
If I try to access the common Accept header key, then I will get the above error.
I think the issue is at: https://github.com/axios/axios/blob/1025d1231a7747503188459dd5a6d1effdcea928/index.d.ts#L3
The type should be:
export type AxiosRequestHeaders = Record<string, Record<string, string>>;
Possibly the AxiosResponseHeaders
has the same issue.
To Reproduce
import axios from 'axios'
axios({
method: 'post',
url: '/user/12345',
data: {
firstName: 'Fred',
lastName: 'Flintstone',
},
transformRequest: (_, headers) => {
if(headers)
delete headers.common['Accept'] //Error occurs here
},
})
.then(function (response) {
console.log(response)
})
.catch(function (error) {
console.log(error)
})
Expected behavior
Expected to not raise an error there
Environment
- Axios 0.23.0
- TypeScript 4.4.4
Additional context/Screenshots
About this issue
- Original URL
- State: closed
- Created 3 years ago
- Reactions: 27
- Comments: 33 (1 by maintainers)
Still having this issue with version
0.26.0
Hey there, any one tries to add
headers
on interceptors will probably have type errors. This because ofheaders
property wasany
previously at version0.21.1
but after0.21.4
till0.22.7
they have added type toheaders
property and it brakes typescript user’s heart.An image from 0.21.1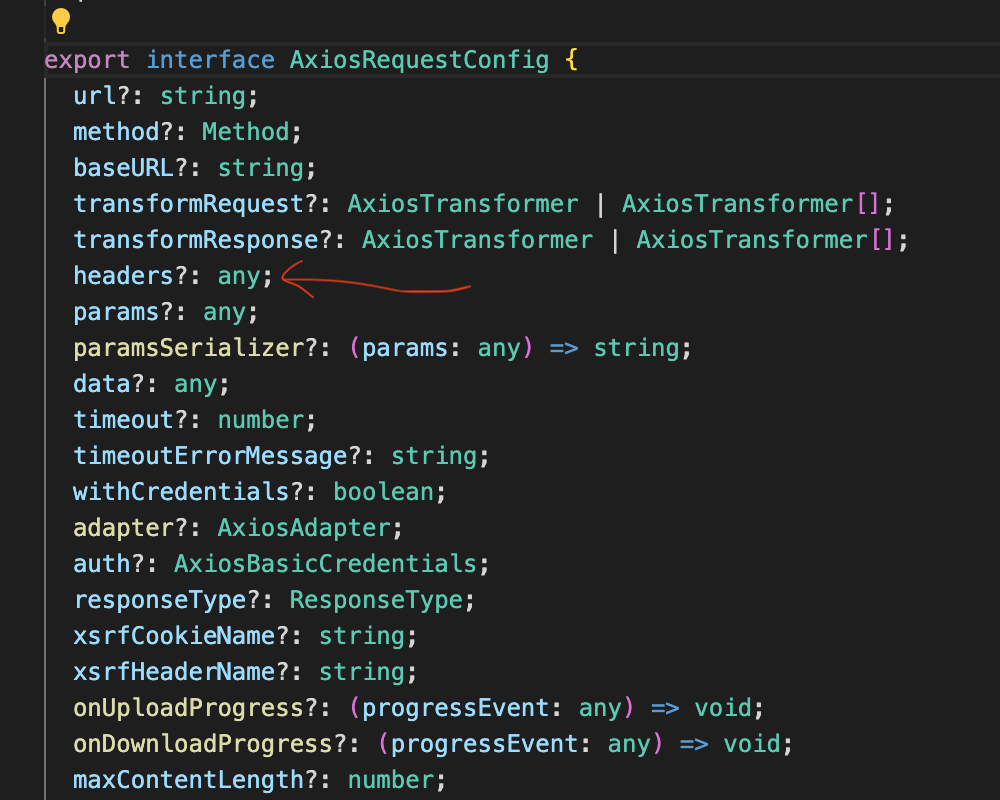
Here is a simple solution
Yep I am still unable to do this in 0.24.0
I am getting
Type 'string | {}' is not assignable to type 'string'. Type '{}' is not assignable to type 'string'.ts(2322)
I have this issue when trying to set the default headers thus:
so setting the
AxiosRequestTransformer
to useHeadersDefaults
wouldn’t fix that?This is still broken in 0.23.0. Our specific use case is that we create instances of axios and want to set the common request headers:
This breaks because in
AxiosRequestConfig
,headers
is now of typeAxiosRequestHeaders
, which is just a type alias forRecord<string, string>
, so it doesn’t allow nested properties. But at this location, nesting incommon
,get
,post
, etc. is perfectly fine.Still having this issue with version
0.25.0
Axios has changed the
defaults
object. Now we can specify defaults per method. To assign to every HTTP method, just use:Example now:
Documentation: https://axios-http.com/docs/config_defaults
I got similar error in version 27.2. My solution was like below.
`import axios, { HeadersDefaults } from ‘axios’;
interface CommonHeaderProps extends HeadersDefaults { “x-validation-key”: string; “x-validation-host”: string; };
axios.defaults.headers = { “x-validation-key”: validationKey, “x-validation-host”: validationHost, } as CommonHeaderProps;`
This should be fixed with the next release, a pre-release should be up a bit later today
Still doesn’t fix the issue. Issue isn’t assigning defaults.
The issue is you cannot define expected response headers (say from an API endpoint with documented header responses that are HeadersDefaults + more stuff)
This line is the issue: https://github.com/axios/axios/blob/ee151a7356ec4498af045dd830312822637890c9/index.d.ts#L115
Fix:
Then in my implementation:
Screenshot in VSCode
I believe the main issue is related to this Omit:
My issue is actually resolved if i use
headers.common
prior to that I was trying to setaxios.defaults.header['thing'] = 'foo';
@Jesus-Escalona your last example works correctly at runtime, it’s only a typing issue. The types need to be fixed accordingly.
+1 on this
May I ask what is the preferred way to instantiate an axios class while setting its headers?
The docs here say it should be done as:
Using this approach I get my custom headers intermingled with the
HeadersDefaults
i.e: common, delete, get, head, post …However, the docs here say one should set the custom header defaults as:
One would think that you could do something like:
But currently getting
object is not assignable to type 'string'.
Note that there is also this example in the README:
In this case the custom header will be at the root of the
headers
object:I am not sure if this is the documentation issue and it should be
headers: { common: 'X-Custom-Header': 'foobar'} }
or a type issue andHeaderDefaults
should also allow arbitrary keys in the root.I think you are right.
HeadersDefaults
would be a better fit for the headers parameter’s type inAxiosRequestTransformer
.Your code at https://github.com/axios/axios/issues/4193#issuecomment-945204765 works. It should would work with the transformers too then. It is also stricter, which is always good.
That’s correct, but it does reference
AxiosRequestHeaders
, which is what causes the issue. Unless I’m misreading the original bug report, one of the effects of the change is to cause an issue with theAxiosRequestTransformer
, but it also causes an issue with any use of theAxiosRequestHeaders
type.With respect, I think there’s a misunderstanding happening here - I’m contending that the issue isn’t specific to
AxiosRequestTransformer
, but all uses of theAxiosRequestHeaders
type, which includesaxios.defaults.headers
+1