react-native-router-flux: Cannot update during an existing state transition
I’m sorry for this newbie question but I can’t understand what’s happening.
This is my code:
import React, { Component } from 'react';
import {
AppRegistry,
StyleSheet,
Text,
Button,
View
} from 'react-native';
import {
Scene,
Router,
Actions
} from 'react-native-router-flux';
class App extends Component {
render() {
return (
<Router>
<Scene key="root">
<Scene key="home" component={Home} title="Home"/>
<Scene key="details" component={Details} title="Details"/>
</Scene>
</Router>
);
}
}
class Home extends Component {
render() {
return (
<View style={styles.container_home}>
<Text style={styles.welcome}>
Home Screen
</Text>
<Button
onPress={()=>Actions.details()}
title="Details"
color="#ffffff"
/>
</View>
);
}
}
class Details extends Component {
render() {
return (
<View style={styles.container_details}>
<Text style={styles.welcome}>
Details Screen
</Text>
</View>
);
}
}
const styles = StyleSheet.create({
container_home: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#333333',
},
container_details: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: 'gray',
},
welcome: {
fontSize: 20,
textAlign: 'center',
margin: 10,
color:'#FFC300'
},
});
AppRegistry.registerComponent('react_native_router_flux', () => App);
And I am getting this message when I save with Hot Reloading:
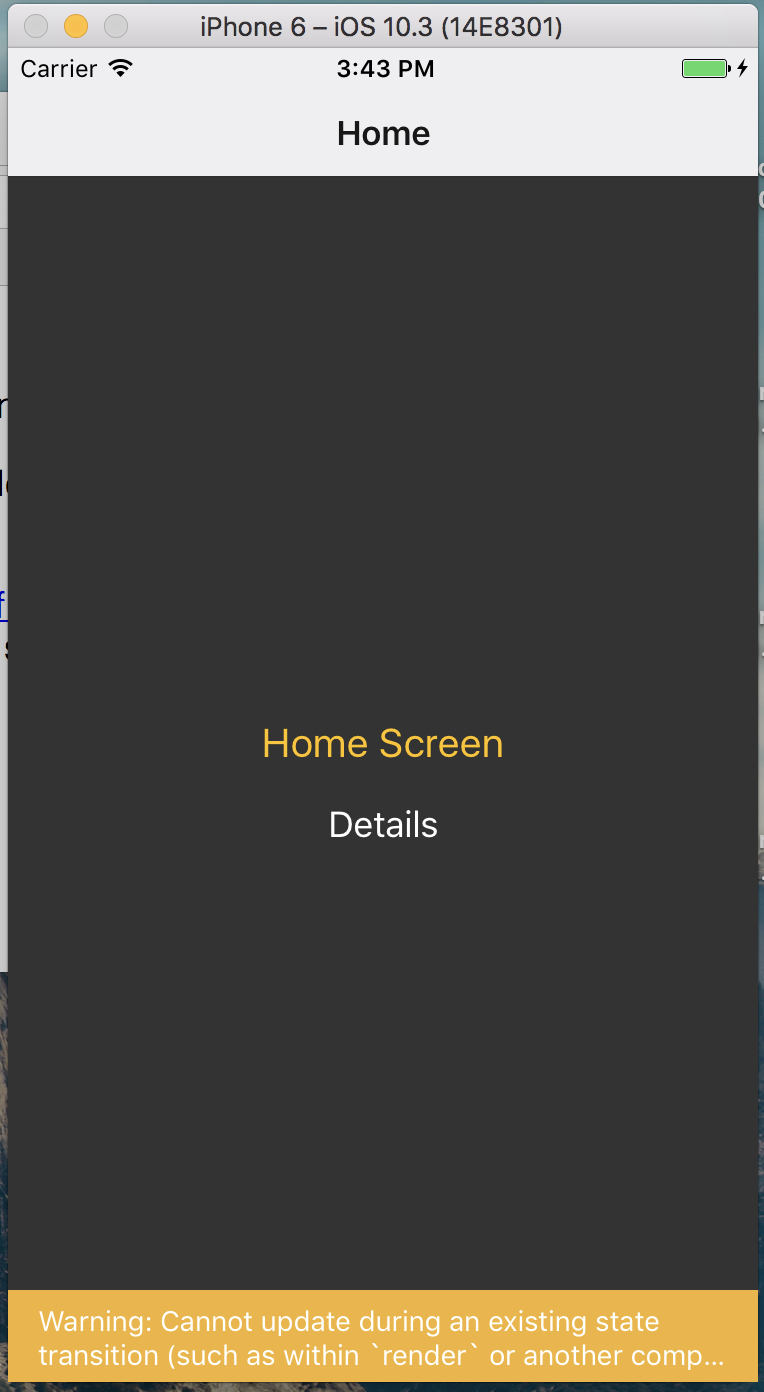
Thanks in advance!
About this issue
- Original URL
- State: closed
- Created 7 years ago
- Reactions: 2
- Comments: 16 (8 by maintainers)
Confirm. There are no warns, if top-level component with Router in render is stateless and rendered only once.
@aksonov would you mind commenting on this? What do you mean with top-level App as stateless component? I’m getting the same error as above and it is coming from mobx form the stacktrace.
@aksonov - I just did a little digging. Would you mind explaining why does the component need to be stateless?
I have an App component that initialises my state, loads the redux store and then passes it to the mainApp which renders the scenes. The mainApp needs to know about part of the state in order to decide which scene to render first. So I’m connecting my mainApp to redux and passing it the part of the state it’s interested in. The second that part of the state changes - I get the error. If I connect my component to redux, but subscribe to an empty state - then no error… Why is it now a problem to have a state? one way around this is to pass the state as Prop to my mainApp like: <Provider store={store}> <mainApp store={store}> </Provider>
Then I can read the part of the state I’m interested in and set which scene to render first, but I don’t understand why is it now no go to connect the router to the state in v4? My code worked fine with v3.
This is working now:
Check react docs about stateless components and check Example project:
const App = () => <Router>...